pthread_t pthread[thread_num];
时间: 2023-12-22 20:28:45 浏览: 41
pthread_t pthread[thread_num];是一个声明了一个pthread_t类型的数组,数组名为pthread,数组大小为thread_num。这个数组可以用来存储多个线程的标识符。
范例:
```c
#include <pthread.h>
int thread_num = 5;
pthread_t pthread[thread_num];
int main() {
// 创建多个线程
for (int i = 0; i < thread_num; i++) {
pthread_create(&pthread[i], NULL, thread_function, NULL);
}
// 等待所有线程结束
for (int i = 0; i < thread_num; i++) {
pthread_join(pthread[i], NULL);
}
return 0;
}
```
相关问题
#include <stdio.h> #include <stdlib.h> #include <pthread.h> #define NUM_THREADS 5 void *thread_func(void *thread_id) { long tid; tid = (long) thread_id; printf("Thread %ld is running\n", tid); // 创建一个新的线程 pthread_t sub_thread; int rc; rc = pthread_create(&sub_thread, NULL, thread_func, (void *) (tid + 1)); if (rc) { printf("Error: return code from pthread_create() is %d\n", rc); exit(-1); } pthread_exit(NULL); } int main(int argc, char *argv[]) { pthread_t threads[NUM_THREADS]; int rc; long t; for (t = 0; t < NUM_THREADS; t++) { printf("Creating thread %ld\n", t); rc = pthread_create(&threads[t], NULL, thread_func, (void *) t); if (rc) { printf("Error: return code from pthread_create() is %d\n", rc); exit(-1); } } // 等待所有线程结束 for (t = 0; t < NUM_THREADS; t++) { pthread_join(threads[t], NULL); } pthread_exit(NULL); }如何在第二个子线程里天机算法
您可以将您的算法代码放在`thread_func`函数中。在您创建新的子线程时,将`thread_func`作为参数传递给`pthread_create`函数,就可以在新的子线程中运行您的算法。以下是修改后的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define NUM_THREADS 5
void *thread_func(void *thread_id) {
long tid;
tid = (long) thread_id;
printf("Thread %ld is running\n", tid);
// 在第二个子线程中添加算法
if (tid == 2) {
printf("Running algorithm in thread %ld\n", tid);
// 这里是您的算法代码
// ...
}
// 创建一个新的子线程
pthread_t sub_thread;
int rc;
rc = pthread_create(&sub_thread, NULL, thread_func, (void *) (tid + 1));
if (rc) {
printf("Error: return code from pthread_create() is %d\n", rc);
exit(-1);
}
pthread_exit(NULL);
}
int main(int argc, char *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
printf("Creating thread %ld\n", t);
rc = pthread_create(&threads[t], NULL, thread_func, (void *) t);
if (rc) {
printf("Error: return code from pthread_create() is %d\n", rc);
exit(-1);
}
}
// 等待所有线程结束
for (t = 0; t < NUM_THREADS; t++) {
pthread_join(threads[t], NULL);
}
pthread_exit(NULL);
}
```
在函数`thread_func`中,当`tid`等于2时,您可以在该线程中运行您的算法。如果您需要访问共享资源,请考虑线程安全问题。
C++ pthread_create
pthread_create是C++中用于创建线程的函数。它是POSIX线程库的一部分,用于在多线程环境下创建新的线程。
pthread_create函数的原型如下:
```c++
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
```
参数说明:
- thread:指向pthread_t类型的指针,用于存储新创建线程的标识符。
- attr:指向pthread_attr_t类型的指针,用于设置线程的属性。可以传入NULL,表示使用默认属性。
- start_routine:指向函数指针,该函数是新线程要执行的函数。函数的返回类型必须为void*,参数类型为void*。
- arg:传递给start_routine函数的参数。
调用pthread_create函数后,会创建一个新的线程,并开始执行start_routine函数。start_routine函数可以是全局函数、静态成员函数或Lambda表达式等。
以下是一个简单的示例代码:
```c++
#include <iostream>
#include <pthread.h>
void* threadFunc(void* arg) {
int* num = static_cast<int*>(arg);
std::cout << "Thread number: " << *num << std::endl;
pthread_exit(NULL);
}
int main() {
pthread_t thread;
int num = 10;
int ret = pthread_create(&thread, NULL, threadFunc, &num);
if (ret != 0) {
std::cerr << "Failed to create thread" << std::endl;
return 1;
}
pthread_join(thread, NULL);
return 0;
}
```
相关推荐
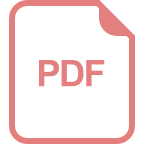
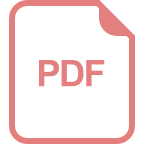
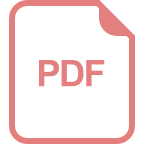












