python实现线性回归算法普通最小二乘法实现过程演示
时间: 2023-08-02 13:07:32 浏览: 112
好的,下面是普通最小二乘法实现线性回归算法的Python代码演示:
```python
import numpy as np
# 构造数据
x_data = np.array([338., 333., 328., 207., 226., 25., 179., 60., 208., 606.])
y_data = np.array([640., 633., 619., 393., 428., 27., 193., 66., 226., 1591.])
# 定义超参数
lr = 0.000001 # 学习率
theta0 = 0. # 初始化theta0
theta1 = 0. # 初始化theta1
epochs = 100000 # 迭代次数
# 定义损失函数
def compute_cost(theta0, theta1, x_data, y_data):
total_cost = np.sum((y_data - theta1 * x_data - theta0) ** 2) / len(y_data)
return total_cost
# 定义梯度下降函数
def gradient_descent(theta0, theta1, x_data, y_data, lr, epochs):
m = len(y_data)
for i in range(epochs):
theta0_grad = 0.
theta1_grad = 0.
for j in range(m):
theta0_grad += (1.0 / m) * ((theta1 * x_data[j] + theta0) - y_data[j])
theta1_grad += (1.0 / m) * ((theta1 * x_data[j] + theta0) - y_data[j]) * x_data[j]
theta0 -= lr * theta0_grad
theta1 -= lr * theta1_grad
if i % 10000 == 0:
print("Epochs: ", i)
print("Cost: ", compute_cost(theta0, theta1, x_data, y_data))
return theta0, theta1
# 运行梯度下降函数
theta0, theta1 = gradient_descent(theta0, theta1, x_data, y_data, lr, epochs)
# 打印训练后的模型参数
print("theta0: ", theta0)
print("theta1: ", theta1)
```
这段代码实现了线性回归算法的普通最小二乘法。首先构造了一组数据(x_data和y_data),然后定义了学习率lr、初始化的theta0和theta1、迭代次数epochs等超参数。接着定义了计算损失函数和梯度下降的函数,最后运行梯度下降函数,输出训练后的模型参数。
阅读全文
相关推荐
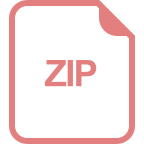
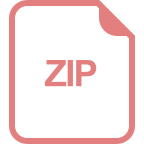
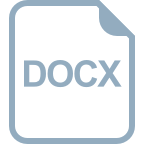















