用python编程:输入一个值用"牛顿迭代法"求平方根。
时间: 2024-10-08 18:22:21 浏览: 19
在Python中,可以使用牛顿迭代法(也叫牛顿-拉夫逊法)来近似计算一个数的平方根。这是一种数值优化算法,通过不断逼近函数的零点来找到方程的解。以下是一个简单的实现步骤:
```python
def sqrt_newton(x, guess=0.0, epsilon=1e-6):
"""
使用牛顿迭代法计算平方根
参数:
x (float): 需要求平方根的数
guess (float): 初始猜测值,默认为0.0
epsilon (float): 容许的误差范围,默认为1e-6
返回:
float: 计算得到的x的平方根
"""
while abs(guess * guess - x) > epsilon:
guess = (guess + x / guess) / 2
return round(guess, 6) # 四舍五入到小数点后6位
# 示例
number = float(input("请输入一个数字: "))
sqrt_result = sqrt_newton(number)
print(f"数字 {number} 的平方根约为: {sqrt_result}")
```
用户需要输入一个数字,程序会使用牛顿迭代法来计算并返回该数的平方根。
相关推荐
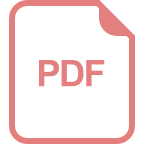
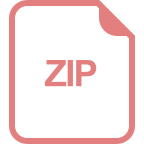
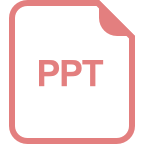
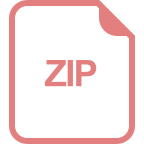
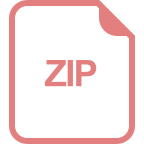
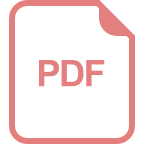
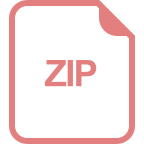
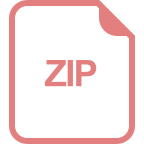
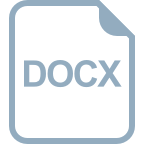