嵌入式Linux如何用C读写can
时间: 2024-04-29 19:25:52 浏览: 156
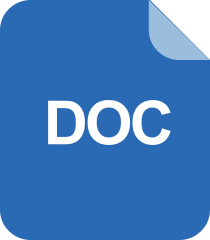
嵌入式Linux_的Cramfs_根文件系统配置成可读可写
嵌入式Linux可以通过SocketCAN接口来读写CAN总线。以下是使用C语言编写的读写CAN的示例代码:
读CAN数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <linux/can.h>
#include <linux/can/raw.h>
int main(void)
{
int s;
struct sockaddr_can addr;
struct ifreq ifr;
struct can_frame frame;
int nbytes;
const char *ifname = "can0";
/* create socket */
if ((s = socket(PF_CAN, SOCK_RAW, CAN_RAW)) < 0) {
perror("socket");
return 1;
}
/* set up can interface */
strcpy(ifr.ifr_name, ifname);
ioctl(s, SIOCGIFINDEX, &ifr);
addr.can_family = AF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
if (bind(s, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("bind");
return 1;
}
/* read data */
while(1) {
nbytes = read(s, &frame, sizeof(struct can_frame));
if (nbytes < 0) {
perror("read");
return 1;
}
printf("ID=%X DLC=%d data=", frame.can_id, frame.can_dlc);
for (int i = 0; i < frame.can_dlc; i++) {
printf("%02X ", frame.data[i]);
}
printf("\n");
}
return 0;
}
```
写CAN数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <linux/can.h>
#include <linux/can/raw.h>
int main(void)
{
int s;
struct sockaddr_can addr;
struct ifreq ifr;
struct can_frame frame;
int nbytes;
const char *ifname = "can0";
/* create socket */
if ((s = socket(PF_CAN, SOCK_RAW, CAN_RAW)) < 0) {
perror("socket");
return 1;
}
/* set up can interface */
strcpy(ifr.ifr_name, ifname);
ioctl(s, SIOCGIFINDEX, &ifr);
addr.can_family = AF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
if (bind(s, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("bind");
return 1;
}
/* write data */
frame.can_id = 0x123;
frame.can_dlc = 8;
frame.data[0] = 0x01;
frame.data[1] = 0x02;
frame.data[2] = 0x03;
frame.data[3] = 0x04;
frame.data[4] = 0x05;
frame.data[5] = 0x06;
frame.data[6] = 0x07;
frame.data[7] = 0x08;
nbytes = write(s, &frame, sizeof(struct can_frame));
if (nbytes < 0) {
perror("write");
return 1;
}
return 0;
}
```
需要注意的是,读写CAN总线需要使用root权限,或者将用户添加到can组中。同时,需要在系统中安装SocketCAN支持,具体安装方法可以参考Linux内核文档中的相关章节。
阅读全文
相关推荐
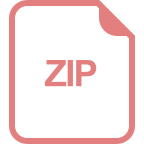
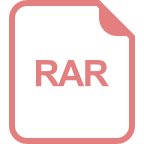
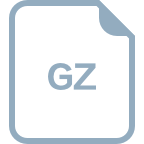
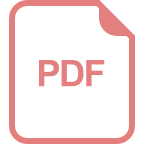
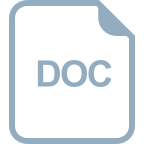

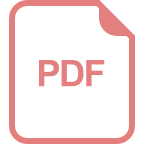
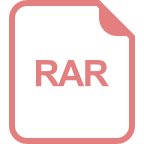
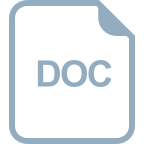
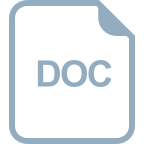
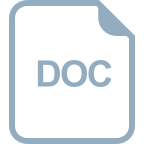
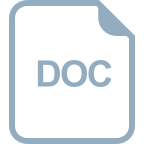
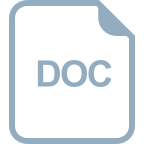
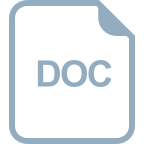
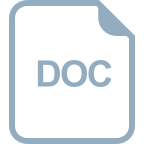