convlstm pytorch
时间: 2023-06-23 20:01:03 浏览: 85
ConvLSTM是一种结合了卷积神经网络和长短期记忆网络的神经网络结构,适用于处理序列数据的预测和生成任务。PyTorch提供了ConvLSTM的实现,可以通过torch.nn.ConvLSTM2d模块来使用。该模块的输入需要是一个四维张量,包含了batch_size、channel、height和width四个维度。你可以通过指定ConvLSTM的输入和隐藏状态的大小、卷积核大小、padding、stride等参数来构建自己的ConvLSTM模型。同时,PyTorch还提供了一些ConvLSTM的变体,如ConvGRU等,可以根据具体任务的需要进行选择使用。
相关问题
ConvLSTM2D pytorch
ConvLSTM2D 是一个基于卷积神经网络和长短期记忆网络 (LSTM) 的模型,用于处理时序数据的预测和建模。在 PyTorch 中,可以使用 torch.nn.ConvLSTM2D 模块来实现 ConvLSTM2D。
下面是一个 ConvLSTM2D 的简单示例代码:
```python
import torch
import torch.nn as nn
# 定义 ConvLSTM2D 模型
class ConvLSTM2DModel(nn.Module):
def __init__(self, input_dim, hidden_dim, kernel_size, num_layers):
super(ConvLSTM2DModel, self).__init__()
self.hidden_dim = hidden_dim
self.num_layers = num_layers
# ConvLSTM2D 层
self.conv_lstm = nn.ConvLSTM2D(input_dim, hidden_dim, kernel_size, num_layers)
# 其他层或操作
...
def forward(self, input):
# 输入形状:(batch_size, seq_len, input_dim, height, width)
# 输出形状:(batch_size, seq_len, hidden_dim, height, width)
output, _ = self.conv_lstm(input)
return output
# 创建 ConvLSTM2D 模型实例
input_dim = 3 # 输入特征维度
hidden_dim = 64 # ConvLSTM2D 隐藏状态维度
kernel_size = (3, 3) # 卷积核大小
num_layers = 2 # ConvLSTM2D 层数
model = ConvLSTM2DModel(input_dim, hidden_dim, kernel_size, num_layers)
# 输入数据示例
input = torch.randn(1, 5, input_dim, 32, 32) # (batch_size, seq_len, input_dim, height, width)
# 模型前向传播
output = model(input)
```
在上述代码中,我们首先定义了一个 ConvLSTM2DModel 类来实现 ConvLSTM2D 模型。在模型的 forward 方法中,我们调用了 nn.ConvLSTM2D 层来进行卷积长短期记忆运算。然后,我们可以创建一个 ConvLSTM2DModel 的实例,将输入数据传递给模型的 forward 方法,即可获得模型的输出结果。
以上是一个基本的 ConvLSTM2D 的 PyTorch 实现示例,你可以根据自己的需求和数据进行相应的调整和扩展。希望对你有所帮助!如果你还有其他问题,请随时提问。
ConvLSTM_pytorch-master
ConvLSTM_pytorch-master是一个用于时间序列预测的深度学习模型。在这个项目中,除了ConvLSTM外还使用了普通LSTM、BiLSTM、Attention LSTM、LightGBM和ARIMA等算法进行预测。您可以在github上找到该项目,具体名称是air_pollutants_prediction_lstm。
另外,为了加速运算,代码中还使用了CUDA。如果您的计算机支持CUDA,可以将数据转换为CUDA张量进行运算。
在作者的github上,您可以找到更多关于该项目的细节以及其他算法的实现。作者也希望能够得到宝贵的意见。
相关推荐
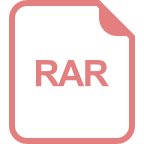
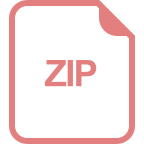












