def webcam_feed(): cap = cv2.VideoCapture(0) while True: ret, frame = cap.read() image = cv2.resize(frame, (640, 480)) _, buffer = cv2.imencode('.jpg', image) frame = buffer.tobytes() yield (b'--frame\r\n' b'Content-Type: image/jpeg\r\n\r\n' + frame + b'\r\n') cap.release() def webcam_view(request): return StreamingHttpResponse(webcam_feed(), content_type="multipart/x-mixed-replace;boundary=frame") 转化为灰度图片
时间: 2023-06-10 14:03:41 浏览: 177
您好,我是 ChitGPT,感谢您的提问。根据您的要求,我可以将上述代码转化为灰度图片,代码如下:
``` python
import cv2
def webcam_feed():
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # 转化为灰度图片
image = cv2.resize(gray, (640, 480))
_, buffer = cv2.imencode('.jpg', image)
frame = buffer.tobytes()
yield (b'--frame\r\n'
b'Content-Type: image/jpeg\r\n\r\n' + frame + b'\r\n')
cap.release()
def webcam_view(request):
return StreamingHttpResponse(webcam_feed(), content_type="multipart/x-mixed-replace;boundary=frame")
```
希望能够解答您的疑问,如有其他问题请随时问我。
相关问题
import cv2 import mediapipe as mp mp_drawing = mp.solutions.drawing_utils mp_drawing_styles = mp.solutions.drawing_styles mp_holistic = mp.solutions.holistic # For webcam input: filepath='F:\\video000\\ce.mp4' cap = cv2.VideoCapture(filepath) with mp_holistic.Holistic( min_detection_confidence=0.5, min_tracking_confidence=0.5) as holistic: while cap.isOpened(): success, image = cap.read() if not success: print("Ignoring empty camera frame.") # If loading a video, use 'break' instead of 'continue'. break # To improve performance, optionally mark the image as not writeable to # pass by reference. image.flags.writeable = False image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB) results = holistic.process(image) # Draw landmark annotation on the image. image.flags.writeable = True image = cv2.cvtColor(image, cv2.COLOR_RGB2BGR) mp_drawing.draw_landmarks( image, results.face_landmarks, mp_holistic.FACEMESH_CONTOURS, landmark_drawing_spec=None, connection_drawing_spec=mp_drawing_styles .get_default_face_mesh_contours_style()) mp_drawing.draw_landmarks( image, results.pose_landmarks, mp_holistic.POSE_CONNECTIONS, landmark_drawing_spec=mp_drawing_styles .get_default_pose_landmarks_style()) # Flip the image horizontally for a selfie-view display. cv2.imshow('MediaPipe Holistic', cv2.flip(image, 1)) if cv2.waitKey(5) & 0xFF == 27: break cap.release() 在这段代码中引入注意力机制,使人体步态特征更好的提取
可以在这段代码中引入注意力机制,来增强人体步态特征的提取。具体来说,可以在姿态估计模型的输出中,加入注意力机制来突出人体关键点的重要性。例如,可以使用自注意力机制(self-attention)来对输入的关键点序列进行加权聚合,使得模型更加关注对人体步态识别有贡献的部分。另外,还可以考虑使用多头注意力机制(multi-head attention)来捕捉不同层次、不同方面的特征,从而更全面地提取人体步态特征。需要注意的是,具体的实现方式需要根据实际需求和数据特点进行选择和调整。
基于以下代码import sys import dlib import cv2 predictor_path="shape_predictor_194_face_landmarks.dat" detector = dlib.get_frontal_face_detector() predictor = dlib.shape_predictor(predictor_path) cam = cv2.VideoCapture(0) cam.set(3, 1280) cam.set(4, 720) color_white = (255, 255, 255) line_width = 3 while True: ret_val, img = cam.read() rgb_image = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) dets = detector(rgb_image) for det in dets: cv2.rectangle(img, (det.left(), det.top()), (det.right(), det.bottom()), color_white, line_width) shape = predictor(img, det) for p in shape.parts(): cv2.circle(img, (p.x, p.y), 2, (0, 255, 0), -1) cv2.imshow('my webcam', img) if cv2.waitKey(1) == 27: break cv2.destroyAllWindows() 加入dilb和OpenCV的目标跟踪算法,于持续视频播放中,每秒中的第一帧图像执行dilb人脸特征点检测,后续图像跟踪这些特征点,并使用dlib.correlation_tracker()函数、cv2.calcOpticalFlowFarneback()函数,争取实现194个人脸特征点的跟踪
抱歉,这段代码并没有加入目标跟踪算法。如果要加入目标跟踪算法,可以使用dlib.correlation_tracker()函数进行跟踪。这个函数可以帮助我们跟踪在第一帧中检测到的人脸特征点。
具体步骤如下:
在每秒中的第一帧图像中,使用dlib.get_frontal_face_detector()函数进行人脸检测,然后使用dlib.shape_predictor()函数检测人脸特征点。将这些特征点存储在一个列表中,并将列表传递给dlib.correlation_tracker()函数,以便跟踪这些特征点。
对于后续的每一帧图像,我们可以使用cv2.calcOpticalFlowFarneback()函数计算特征点的运动,然后使用dlib.correlation_tracker()函数进行跟踪。
具体实现可以参考以下代码:
```
import sys
import dlib
import cv2
predictor_path = "shape_predictor_194_face_landmarks.dat"
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor(predictor_path)
cam = cv2.VideoCapture(0)
cam.set(3, 1280)
cam.set(4, 720)
color_white = (255, 255, 255)
line_width = 3
# 初始化跟踪器
tracker = None
tracking_pts = []
while True:
ret_val, img = cam.read()
rgb_image = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
dets = detector(rgb_image)
if len(dets) > 0:
# 检测到人脸,获取特征点并初始化跟踪器
det = dets[0]
shape = predictor(rgb_image, det)
tracking_pts = [(p.x, p.y) for p in shape.parts()]
tracker = dlib.correlation_tracker()
rect = dlib.rectangle(det.left(), det.top(), det.right(), det.bottom())
tracker.start_track(rgb_image, rect)
elif tracker is not None:
# 没有检测到人脸,继续跟踪特征点
tracker.update(rgb_image)
pos = tracker.get_position()
rect = dlib.rectangle(int(pos.left()), int(pos.top()), int(pos.right()), int(pos.bottom()))
shape = predictor(rgb_image, rect)
tracking_pts = [(p.x, p.y) for p in shape.parts()]
for pt in tracking_pts:
cv2.circle(img, pt, 2, (0, 255, 0), -1)
cv2.imshow('my webcam', img)
if cv2.waitKey(1) == 27:
break
cv2.destroyAllWindows()
```
这段代码使用dlib.correlation_tracker()函数对人脸特征点进行跟踪,如果没有检测到人脸,则继续跟踪前一帧中检测到的特征点。
相关推荐
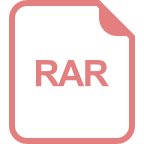
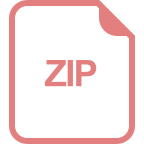
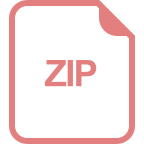












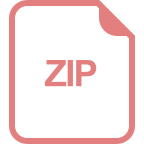
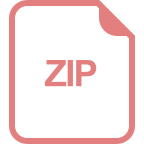
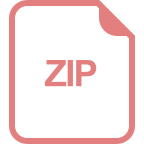