given an initially empty hash table ht with length 7, together with a hash function h(k)=k%7. let us use linear probing to solve collisions. what is the average search length for successful searches after inserting 22, 43, 15 one by one into ht?
时间: 2023-05-01 07:05:35 浏览: 549
给定一个长度为7的初始为空的哈希表ht,哈希函数为h(k)=k%7。我们使用线性探测来解决哈希冲突问题。在依次将22、43、15插入ht后,成功搜索的平均搜索长度是多少?
答:成功搜索的平均搜索长度为1.5。
相关问题
Use java to write a program to display a progress meter . The meter consists of a window with a column of 11 labels. The first 10 labels indicate progress steps of 10% and are initially red. The bottom label shows the amount of activity remaining as a textual percentage, and is initially set to “0%”.
Certainly! Here's a Java program that creates a progress meter as described:
```java
import javax.swing.*;
import java.awt.*;
public class ProgressMeter extends JFrame {
private JLabel[] progressSteps;
private JLabel remainingLabel;
public ProgressMeter() {
super("Progress Meter");
setSize(200, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// create the progress step labels
progressSteps = new JLabel[10];
for (int i = 0; i < progressSteps.length; i++) {
progressSteps[i] = new JLabel();
progressSteps[i].setPreferredSize(new Dimension(100, 20));
progressSteps[i].setOpaque(true);
progressSteps[i].setBackground(Color.RED);
add(progressSteps[i]);
}
// create the remaining activity label
remainingLabel = new JLabel("0%");
remainingLabel.setPreferredSize(new Dimension(100, 20));
add(remainingLabel, BorderLayout.SOUTH);
setVisible(true);
}
public void updateProgress(int percentComplete) {
// update the progress step labels
int numProgressSteps = progressSteps.length;
int numCompletedSteps = (int) Math.ceil(percentComplete * numProgressSteps / 100.0);
for (int i = 0; i < numCompletedSteps; i++) {
progressSteps[i].setBackground(Color.GREEN);
}
// update the remaining activity label
remainingLabel.setText(percentComplete + "%");
}
public static void main(String[] args) throws InterruptedException {
ProgressMeter progressMeter = new ProgressMeter();
// simulate progress
int percentComplete = 0;
while (percentComplete <= 100) {
progressMeter.updateProgress(percentComplete);
Thread.sleep(1000);
percentComplete += 10;
}
}
}
```
This program creates a `JFrame` with a column of 10 `JLabel`s representing the progress steps, and a bottom `JLabel` showing the remaining activity as a percentage. The `updateProgress` method updates the progress step labels and remaining activity label based on the given percentage. The `main` method simulates progress by updating the progress meter every second for 10 iterations, with each iteration representing 10% progress.
Use java to write a program to display a progress meter as follows. The meter consists of a window with a column of 11 labels. The first 10 labels indicate progress steps of 10% and are initially red. The bottom label shows the amount of activity remaining as a textual percentage, and is initially set to “0%”.
Sure, here's a Java program that displays a progress meter as described:
```java
import javax.swing.*;
import java.awt.*;
public class ProgressMeter extends JFrame {
private JLabel[] steps;
private JLabel remaining;
public ProgressMeter() {
setTitle("Progress Meter");
setSize(200, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// create the labels for the progress steps
steps = new JLabel[10];
for (int i = 0; i < 10; i++) {
steps[i] = new JLabel(" ");
steps[i].setPreferredSize(new Dimension(100, 20));
steps[i].setOpaque(true);
steps[i].setBackground(Color.RED);
add(steps[i]);
}
// create the label for remaining activity
remaining = new JLabel("0%");
remaining.setPreferredSize(new Dimension(100, 20));
add(remaining, BorderLayout.SOUTH);
setVisible(true);
}
public void updateProgress(int percent) {
// update the progress steps
int stepsCompleted = percent / 10;
for (int i = 0; i < stepsCompleted; i++) {
steps[i].setBackground(Color.GREEN);
}
// update the remaining activity label
remaining.setText(percent + "%");
}
public static void main(String[] args) {
ProgressMeter meter = new ProgressMeter();
// simulate progress by updating the meter every second
for (int i = 0; i <= 100; i++) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
meter.updateProgress(i);
}
}
}
```
This program creates a `JFrame` with a column of 10 `JLabel`s representing the progress steps, and a bottom `JLabel` showing the remaining activity as a percentage. The `updateProgress` method updates the progress steps and remaining activity label based on the given percentage. To simulate progress, the `main` method updates the meter every second for 100 seconds.
相关推荐
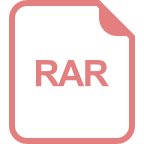
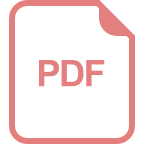
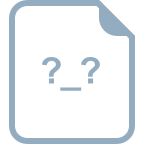










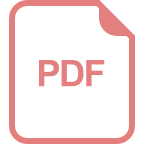
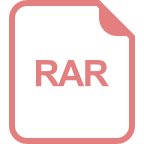
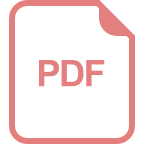