蚁群算法旅行商问题Python
时间: 2023-07-27 08:07:07 浏览: 57
蚁群算法可以用来解决旅行商问题,下面是一个Python实现的例子:
```python
import random
class Ant:
def __init__(self, num_cities):
self.position = random.randint(0, num_cities-1)
self.visited = [False] * num_cities
self.visited[self.position] = True
self.tour_length = 0
def move(self, pheromone, alpha, beta):
next_city = self.select_next_city(pheromone, alpha, beta)
self.visited[next_city] = True
self.tour_length += pheromone[self.position][next_city]
self.position = next_city
def select_next_city(self, pheromone, alpha, beta):
total = 0
probs = []
unvisited_cities = [i for i, visited in enumerate(self.visited) if not visited]
for city in unvisited_cities:
total += pheromone[self.position][city] ** alpha * ((1.0 / distance_matrix[self.position][city]) ** beta)
for city in unvisited_cities:
prob = pheromone[self.position][city] ** alpha * ((1.0 / distance_matrix[self.position][city]) ** beta) / total
probs.append((city, prob))
probs.sort(key=lambda x: x[1], reverse=True)
return probs[0][0]
class ACO:
def __init__(self, num_ants, num_iterations, alpha, beta, rho, q):
self.num_ants = num_ants
self.num_iterations = num_iterations
self.alpha = alpha
self.beta = beta
self.rho = rho
self.q = q
def solve(self, distance_matrix):
pheromone = [[1.0/distance_matrix[i][j] for j in range(len(distance_matrix))] for i in range(len(distance_matrix))]
best_tour = None
best_tour_length = float('inf')
for iteration in range(self.num_iterations):
ants = [Ant(len(distance_matrix)) for i in range(self.num_ants)]
for ant in ants:
for i in range(len(distance_matrix)-1):
ant.move(pheromone, self.alpha, self.beta)
ant.tour_length += distance_matrix[ant.position][ants[0].position]
if ant.tour_length < best_tour_length:
best_tour_length = ant.tour_length
best_tour = ant.visited[:]
for i in range(len(distance_matrix)):
pheromone[ant.position][i] *= (1 - self.rho)
for i in range(len(distance_matrix)-1):
pheromone[ant.visited[i]][ant.visited[i+1]] += self.q / ant.tour_length
pheromone[ant.visited[-1]][ants[0].position] += self.q / ant.tour_length
return best_tour, best_tour_length
```
其中,Ant类表示一个蚂蚁,ACO类实现了蚁群算法的主体逻辑。在ACO类中,solve方法接受一个距离矩阵作为输入,并返回最佳路径和路径长度。在solve方法中,我们首先初始化pheromone矩阵,然后执行num_iterations轮迭代。在每一轮迭代中,我们创建num_ants个蚂蚁,让它们走一遍路径,并更新pheromone矩阵。在更新pheromone矩阵时,我们先让所有的pheromone值递减(即挥发),然后让每只蚂蚁根据经过的路径增加pheromone值。
下面是一个使用该算法求解旅行商问题的例子:
```python
# 生成随机距离矩阵
num_cities = 10
distance_matrix = [[random.randint(1, 100) for j in range(num_cities)] for i in range(num_cities)]
# 调用蚁群算法求解
aco = ACO(num_ants=50, num_iterations=100, alpha=1, beta=5, rho=0.1, q=100)
best_tour, best_tour_length = aco.solve(distance_matrix)
# 输出结果
print("Best tour:", best_tour)
print("Best tour length:", best_tour_length)
```
在上面的例子中,我们生成了一个10x10的随机距离矩阵,并使用ACO类求解旅行商问题。ACO类的参数可以根据实际问题进行调整。最后,我们输出了最佳路径和路径长度。
相关推荐
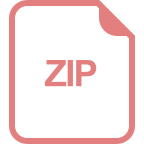
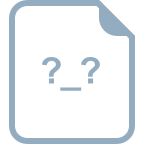














