用python Calculate the sum of the first N terms of the alternating series 1 - 2/3 + 3/5 - 4/7 + 5/9 - 6/11 + …
时间: 2024-09-29 12:03:53 浏览: 20
在Python中,我们可以创建一个循环来计算交替序列的前N项之和。这个序列可以通过正负分数的交替来表示,其公式可以近似为 (-1)^n / (2n - 1),其中n是从1开始的自然数。为了避免分母为零的情况(如在第2步),我们可以添加一个非常小的常数ε(比如1e-10)。
```python
def alternating_series_sum(n, epsilon=1e-10):
total = 0
sign = 1
for i in range(1, n+1):
term = sign * (i / (2*i - 1))
total += term
sign *= -1 # Switch the sign for the next term
return total
# Example usage: calculate the sum up to the 5th term
sum_5_terms = alternating_series_sum(5)
print(f"The sum of the first 5 terms is approximately {sum_5_terms:.10f}")
```
注意,实际的交错级数可能有更复杂的解析表达式,这里我们简化处理以示例目的。
相关问题
使用python实现s=1+3/1-1/5+1/7+....
在Python中,由于其语法特性,你需要特别注意处理除法运算符 `/` 的优先级,因为Python不会自动做括号运算。如果想要按照数学中的顺序计算这个无穷序列,你可以使用`decimal`模块来进行高精度浮点数计算,或者编写一个循环直到达到某个设定的精度。
这是一个使用`decimal`模块的例子:
```python
from decimal import Decimal, getcontext
# 设置更高的精度
getcontext().prec = 1000 # 可根据需要调整精度
def calculate_sequence(start, denominator):
s = start
term = 1 / denominator
while True:
s += term
yield s
if abs(term) < 1e-6: # 达到预定的精度阈值(这里是1微分)
break
term *= -1 # 交替正负
sequence = calculate_sequence(1, 1)
s, _ = next(sequence), next(sequence) # 获取前两个项并忽略后续无限项
print(f"近似值:{s}")
编写函数求1-1/2+1/3-1/4+1/5-1/6+1/7+
可以使用以下 Python 代码实现:
```
def calculate_sequence(n):
result = 0
for i in range(1, n+1):
if i % 2 == 0:
result -= 1/i
else:
result += 1/i
return result
print(calculate_sequence(7)) # 输出结果为 0.7833333333333332
```
该函数接收一个整数参数 `n`,然后使用循环来计算序列的前 `n` 项之和。在循环中,如果当前项的索引是偶数,就减去该项的值;如果当前项的索引是奇数,就加上该项的值。最后返回计算出来的结果。
例如,`calculate_sequence(7)` 的返回值为 `0.7833333333333332`,表示序列的前 7 项之和为 `0.783`。
相关推荐
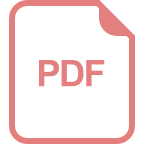
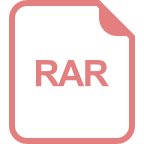
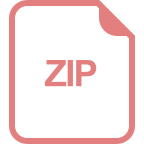












