用c++完成,已知从键盘输入三个数作为三角形的三边,在输入正确的情况下计算三角形的面积并输出到屏幕上,如果用户输入某边为0则抛出ZeroException异常,并输出提示the divisor is zero!,如果用户输入某边为负数,则抛出InputException,并输出提示the input number is negative error!,如果输入三个数无法构成三角形,比如两边之和大于第三边,或者两边之差小于第三边,则抛出NoTriangException异常,输出"Three numbers cannot form a triangle!"。
时间: 2024-03-20 13:44:24 浏览: 130
好的,这是一个计算三角形面积的程序,但要处理多种异常情况。下面是示例代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class ZeroException : public exception {
public:
const char* what() const throw() {
return "the divisor is zero!";
}
};
class InputException : public exception {
public:
const char* what() const throw() {
return "the input number is negative error!";
}
};
class NoTriangException : public exception {
public:
const char* what() const throw() {
return "Three numbers cannot form a triangle!";
}
};
double calcTriangleArea(double a, double b, double c) {
if (a <= 0 || b <= 0 || c <= 0) {
throw InputException();
}
if (a + b <= c || a + c <= b || b + c <= a) {
throw NoTriangException();
}
double p = (a + b + c) / 2;
double area = sqrt(p * (p - a) * (p - b) * (p - c));
return area;
}
int main() {
double a, b, c;
try {
cout << "请输入三角形的三边:";
cin >> a >> b >> c;
double area = calcTriangleArea(a, b, c);
cout << "三角形的面积为:" << area << endl;
} catch (ZeroException& e) {
cout << e.what() << endl;
} catch (InputException& e) {
cout << e.what() << endl;
} catch (NoTriangException& e) {
cout << e.what() << endl;
} catch (...) {
cout << "发生了未知错误!" << endl;
}
return 0;
}
```
以上代码中,我们定义了三个异常类:ZeroException 表示输入了零,InputException 表示输入了负数,NoTriangException 表示输入的三条边无法构成三角形。在 `calcTriangleArea()` 函数中,我们使用 `throw` 语句抛出这些异常;在 `main()` 函数中,我们使用 `try-catch` 语句块捕获这些异常并进行处理。如果没有异常,则计算三角形面积并输出;如果有异常,则输出对应的提示信息。
阅读全文
相关推荐



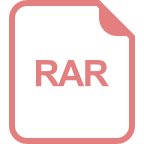














