如何使用python实现对DM二维码的矫正,并计算输出其中心坐标,偏移角度和二维码内容的0-1矩阵
时间: 2024-06-11 09:06:50 浏览: 273
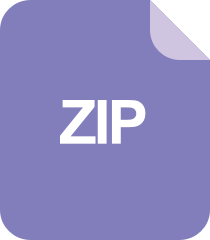
Python+OpenCV查找二维码图片中的定位图案 回字形图案

实现对DM二维码的矫正及计算其中心坐标、偏移角度和二维码内容的0-1矩阵,可以通过以下步骤实现:
步骤1:导入相关库
```python
import cv2
import numpy as np
from pylibdmtx.pylibdmtx import decode
```
步骤2:读取二维码图像并进行灰度处理
```python
img = cv2.imread('dm_code.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
步骤3:使用边缘检测算法提取二维码轮廓
```python
edges = cv2.Canny(gray, 100, 200)
contours, hierarchy = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
步骤4:寻找二维码的外接矩形并进行矫正
```python
rect = cv2.minAreaRect(contours[0])
box = cv2.boxPoints(rect)
box = np.int0(box)
width = int(rect[1][0])
height = int(rect[1][1])
src_pts = box.astype("float32")
dst_pts = np.array([[0, height-1],
[0, 0],
[width-1, 0],
[width-1, height-1]], dtype="float32")
M = cv2.getPerspectiveTransform(src_pts, dst_pts)
warped = cv2.warpPerspective(img, M, (width, height))
```
步骤5:计算二维码中心坐标
```python
center_x = int(rect[0][0])
center_y = int(rect[0][1])
```
步骤6:计算二维码偏移角度
```python
angle = rect[2]
if angle < -45:
angle = 90 + angle
```
步骤7:解码二维码内容并输出0-1矩阵
```python
decoded = decode(warped)
data = decoded[0].data.decode('utf-8')
matrix = decoded[0].to_array()
print('二维码内容:', data)
print('0-1矩阵:')
print(matrix)
```
完整代码如下:
```python
import cv2
import numpy as np
from pylibdmtx.pylibdmtx import decode
# 读取图像并进行灰度处理
img = cv2.imread('dm_code.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 边缘检测提取轮廓
edges = cv2.Canny(gray, 100, 200)
contours, hierarchy = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 矫正二维码
rect = cv2.minAreaRect(contours[0])
box = cv2.boxPoints(rect)
box = np.int0(box)
width = int(rect[1][0])
height = int(rect[1][1])
src_pts = box.astype("float32")
dst_pts = np.array([[0, height-1],
[0, 0],
[width-1, 0],
[width-1, height-1]], dtype="float32")
M = cv2.getPerspectiveTransform(src_pts, dst_pts)
warped = cv2.warpPerspective(img, M, (width, height))
# 计算中心坐标和偏移角度
center_x = int(rect[0][0])
center_y = int(rect[0][1])
angle = rect[2]
if angle < -45:
angle = 90 + angle
# 解码二维码并输出0-1矩阵
decoded = decode(warped)
data = decoded[0].data.decode('utf-8')
matrix = decoded[0].to_array()
print('二维码内容:', data)
print('0-1矩阵:')
print(matrix)
```
阅读全文
相关推荐
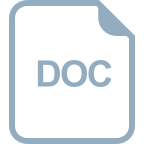
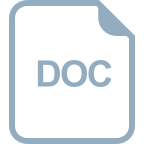
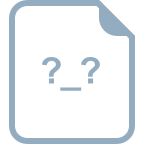
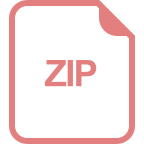
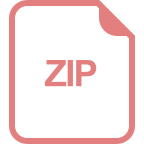
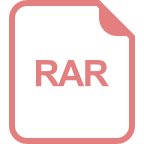
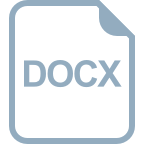
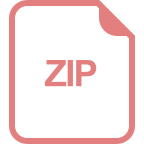
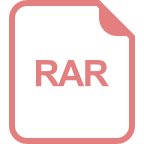
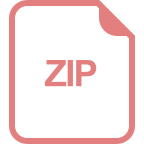
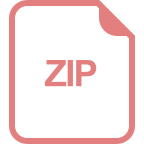
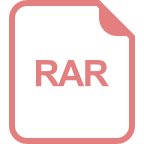
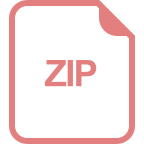
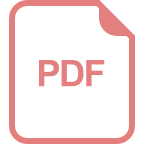
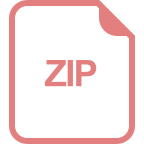
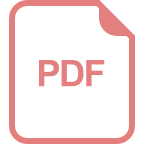