torch flatten.transpose
时间: 2023-10-15 22:22:40 浏览: 50
在PyTorch中,flatten函数用于将张量展平为一维。transpose函数则用于交换张量的维度。
如果要将一个张量展平为一维,并且交换其维度,则可以按照以下方式使用flatten和transpose函数:
```python
import torch
# 创建一个二维张量
tensor = torch.tensor([[1, 2, 3], [4, 5, 6]])
# 使用flatten函数展平张量为一维
flattened_tensor = tensor.flatten()
print(flattened_tensor)
# 使用transpose函数交换张量的维度
transposed_tensor = flattened_tensor.transpose(0, 1)
print(transposed_tensor)
```
输出结果为:
```
tensor([1, 2, 3, 4, 5, 6])
tensor([1, 2, 3, 4, 5, 6])
```
注意,由于我们的输入张量已经是一维的,所以transpose函数不会对其产生任何效果。
相关问题
class MotionEncoder_STGCN(nn.Module): def __init__(self): super(MotionEncoder_STGCN, self).__init__() self.graph_args = {} self.st_gcn = ST_GCN(in_channels=2, out_channels=32, graph_args=self.graph_args, edge_importance_weighting=True, mode='M2S') self.fc = nn.Sequential(nn.Conv1d(32 * 13, 64, kernel_size=1), nn.BatchNorm1d(64)) def forward(self, input): input = input.transpose(1, 2) input = input.transpose(1, 3) input = input.unsqueeze(4) output = self.st_gcn(input) output = output.transpose(1, 2) output = torch.flatten(output, start_dim=2) output = self.fc(output.transpose(1, 2)).transpose(1, 2) return output def features(self, input): input = input.transpose(1, 2) input = input.transpose(1, 3) input = input.unsqueeze(4) output = self.st_gcn(input) output = output.transpose(1, 2) output = torch.flatten(output, start_dim=2) output = self.fc(output.transpose(1, 2)).transpose(1, 2) features = self.st_gcn.extract_feature(input) features.append(output.transpose(1, 2)) return features
这是一个 Python 代码段,用于定义一个名为 MotionEncoder_STGCN 的类,该类包含一个前向传递函数和一个特征提取函数。它使用 ST_GCN 模型对输入进行处理,并使用卷积神经网络对输出进行处理。我可以回答这个问题。
def channel_shuffle(x, groups): batchsize, num_channels, height, width = x.data.size() channels_per_group = num_channels // groups # reshape x = x.view(batchsize, groups, channels_per_group, height, width) x = torch.transpose(x, 1, 2).contiguous() # flatten x = x.view(batchsize, -1, height, width) return x
这是一个用于通道混洗的函数。输入参数 x 是一个四维的张量,表示一个 batch 内的多张图片;groups 表示要分成的组数。
函数首先从输入张量 x 中获取 batch size、channel 数量、高度和宽度四个维度的大小。然后计算出每组应当包含的 channel 数量,即 channels_per_group = num_channels // groups。
接着,函数将输入张量 x reshape 成新的形状,使得通道数按照 groups 和 channels_per_group 进行划分。具体来说,新的形状为 batchsize * groups * channels_per_group * height * width,其中第二个维度是 groups,第三个维度是 channels_per_group。
接下来,函数使用 torch.transpose() 函数将第二个和第三个维度进行交换,以实现通道混洗的效果。注意,由于交换维度后张量的存储顺序可能不再是连续的,因此需要调用 .contiguous() 方法,使得张量在内存中是连续存储的。
最后,函数将张量再次 reshape,将前两个维度合并为一个,即 batchsize * (groups * channels_per_group) * height * width,并返回结果。
相关推荐
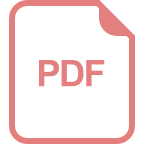












