JavaScript:Complete the solution so that it splits the string into pairs of two characters. If the string contains an odd number of characters then it should replace the missing second character of the final pair with an underscore ('_'). Examples: * 'abc' => ['ab', 'c_'] * 'abcdef' => ['ab', 'cd', 'ef']
时间: 2023-12-25 09:04:51 浏览: 358
Sure! Here's a JavaScript function that solves the problem you described:
```javascript
function splitStringIntoPairs(str) {
var pairs = [];
for (var i = 0; i < str.length; i += 2) {
var pair = str.slice(i, i + 2);
if (pair.length < 2) {
pair += '_';
}
pairs.push(pair);
}
return pairs;
}
console.log(splitStringIntoPairs('abc')); // Output: ['ab', 'c_']
console.log(splitStringIntoPairs('abcdef')); // Output: ['ab', 'cd', 'ef']
```
The `splitStringIntoPairs` function takes a string as input and iterates over it, extracting pairs of two characters. If the length of the pair is less than 2 (i.e., odd number of characters), it appends an underscore ('_') to the pair. Finally, it returns an array of the pairs.
Hope this helps! Let me know if you have any other questions.
阅读全文
相关推荐
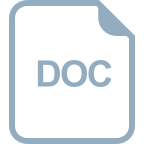
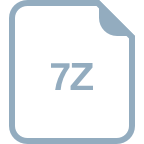
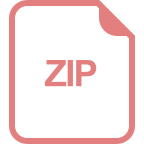
















