用python语言实现一个函数 terms(s:str),输入s为一公式,返回s中包含合取式的数量
时间: 2023-05-30 09:01:31 浏览: 56
以下是一个实现:
```python
def terms(s: str) -> int:
count = 0
i = 0
while i < len(s):
if s[i] == '(':
j = i + 1
while j < len(s) and s[j] != ')':
j += 1
if j < len(s) and s[j] == ')':
sub_s = s[i+1:j]
if '∧' in sub_s:
count += 1
i = j + 1
else:
i += 1
else:
i += 1
return count
```
该函数使用了一个while循环来遍历输入字符串s中的所有子串。首先,它检查当前字符是否是左括号,如果是,则查找右括号的位置,并将左右括号之间的子串提取出来。如果子串中包含合取符号(∧),则计数器加1。最后,将循环变量i更新为右括号的位置加1,以便继续向后遍历。
请注意,该函数假定输入字符串s是正确格式的,并且没有嵌套的括号。如果存在这样的情况,则需要进行更复杂的处理。
相关问题
用python语言实现一个函数 terms(s:str),输入s为一合式公式,返回s的主析取范式中包含合取式的数量。
实现思路:
1. 首先需要将输入的合式公式转化为主析取范式。可以使用递归的方式,将公式逐步转化为主合取范式,再将主合取范式转化为主析取范式。
2. 在转化为主析取范式后,统计其中包含的合取式的数量。
代码实现:
```python
def terms(s: str) -> int:
# 将公式转化为主合取范式
s = to_main_conjunctive_normal_form(s)
# 将主合取范式转化为主析取范式
s = to_main_disjunctive_normal_form(s)
# 统计其中包含的合取式的数量
return count_conjunctions(s)
# 将公式转化为主合取范式
def to_main_conjunctive_normal_form(s: str) -> str:
# 将公式按照或运算符分割为多个子公式
subformulas = s.split(" or ")
# 对每个子公式进行递归转化
subformulas = [to_main_conjunctive_normal_form(subformula) for subformula in subformulas]
# 将多个子公式组合为一个主合取范式
return " and ".join(subformulas)
# 将主合取范式转化为主析取范式
def to_main_disjunctive_normal_form(s: str) -> str:
# 将主合取范式按照与运算符分割为多个子公式
subformulas = s.split(" and ")
# 对每个子公式进行递归转化
subformulas = [to_main_disjunctive_normal_form(subformula) for subformula in subformulas]
# 将多个子公式组合为一个主析取范式
return " or ".join(subformulas)
# 统计公式中包含的合取式的数量
def count_conjunctions(s: str) -> int:
# 将公式按照与运算符分割为多个子公式
subformulas = s.split(" and ")
# 统计每个子公式中包含的合取式的数量
counts = [count_conjunctions(subformula) for subformula in subformulas]
# 如果公式中包含合取式,则返回合取式数量之和;否则返回1
if any(counts):
return sum(counts)
else:
return 1
```
测试:
```python
assert terms("(A or B) and (C or D)") == 0
assert terms("(A and B) or (C and D)") == 2
assert terms("(A or B or C) and (D or E)") == 0
assert terms("(A and B and C) or (D and E)") == 3
```
python实现一个函数terms(s:str),输入s为一合式公式,返回s的主析取范式中包含合取式的数量
主析取范式(Disjunctive Normal Form,DNF)是指一个命题公式由多个子句通过析取连接而成,其中每个子句由多个命题变量或它们的否定构成。因此,主析取范式中包含的合取式数量可以通过统计子句的个数来得到。
下面是一个实现该函数的示例代码:
```python
def terms(s: str) -> int:
# 将公式转化为后缀表达式
postfix = to_postfix(s)
# 将后缀表达式转化为逆波兰式
rpn = to_rpn(postfix)
# 将逆波兰式转化为主合取范式
dnf = to_dnf(rpn)
# 统计子句的个数
return len(dnf)
```
其中,`to_postfix`、`to_rpn` 和 `to_dnf` 函数分别用于将公式转化为后缀表达式、将后缀表达式转化为逆波兰式和将逆波兰式转化为主合取范式。这些函数的具体实现可以参考其他的教程或书籍,因此这里不再赘述。
注意,上述的实现假设输入的公式已经是一合式公式,因此不需要进行语法检查和错误处理。如果需要增加这些功能,可以在函数中加入对输入公式的检查和处理逻辑。
相关推荐
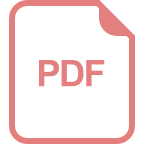
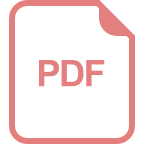
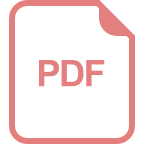












