implements和using
时间: 2024-11-09 08:25:28 浏览: 13
"implements" 和 "using" 都是在编程中使用的关键字,但它们代表的概念有所不同:
1. "implements": 这是一个在面向对象编程语言(如Java)中用于实现接口的关键字。当你声明一个类实现了某个接口时,意味着这个类需要提供接口中所列的所有方法的实现。例如:
```java
public class MyClass implements MyInterface {
// 实现MyInterface的方法...
}
```
这样,`MyClass` 就有了接口的功能,可以被当作接口的实例来使用。
2. "using": 这个术语通常在C#中出现,它表示命名空间(Namespace)的导入。通过 `using` 关键字,你可以引入其他命名空间中的类、结构体或接口,以便在当前文件中直接使用它们而无需写出完整的命名空间路径。例如:
```csharp
using System;
// 现在可以直接使用System.IO命名空间下的类
class MyClass {
void SomeMethod() {
Console.WriteLine("Hello");
}
}
```
相关问题
implements Serializable
The "implements Serializable" statement is used to indicate that a class in Java can be serialized, which means it can be converted into a stream of bytes that can be saved to a file or sent over a network. This allows the object to be reconstructed later, possibly on a different machine or at a different time, while preserving its state.
To implement serialization, a class needs to implement the Serializable interface, which is a marker interface with no methods. This means that the class only needs to declare that it implements Serializable, and the serialization mechanism will automatically handle the rest.
Here is an example of a class that implements Serializable:
```
public class Person implements Serializable {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
In this example, the Person class has two fields (name and age) and a constructor and two getter methods. By implementing Serializable, this class can now be serialized and deserialized using Java's built-in serialization mechanism.
Java implements
many different features and concepts, including:
1. Object-oriented programming: Java is an object-oriented programming language, which means that it provides support for creating and using objects.
2. Garbage collection: Java automatically manages memory allocation and deallocation through a process known as garbage collection.
3. Platform-independent: Java is platform-independent, meaning that it can run on any platform that has a Java Virtual Machine (JVM) installed.
4. Multithreading: Java provides support for multithreading, which allows multiple threads of execution to run concurrently within a program.
5. Exception handling: Java provides a robust exception handling mechanism that allows programmers to handle errors and exceptions in a structured and controlled way.
6. Generics: Java uses generics to provide type safety and overcome the limitations of using raw types.
7. Annotations: Java provides support for annotations, which allow programmers to add metadata to code and provide additional information to the compiler.
8. Reflection: Java allows programmers to inspect and modify the behavior of objects and classes at runtime through a feature called reflection.
9. Lambda expressions: Java 8 introduced lambda expressions, which provide a concise and expressive way to write code using functional programming concepts.
10. Streams: Java 8 also introduced streams, which provide a powerful and efficient way to process collections of data.
阅读全文
相关推荐



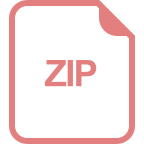













