请给我一个俄罗斯方块的py代码
时间: 2024-02-01 11:17:09 浏览: 79
以下是一个简单的俄罗斯方块的 Python 代码,使用 Pygame 库实现:
```python
import pygame
import random
# 游戏区域大小
SCREEN_WIDTH = 300
SCREEN_HEIGHT = 600
# 方块大小
BLOCK_SIZE = 30
# 方块类型及形状
BLOCKS = [
[[1, 1, 1], [0, 1, 0]],
[[0, 2, 2], [2, 2, 0]],
[[3, 3, 0], [0, 3, 3]],
[[4, 0, 0], [4, 4, 4]],
[[0, 0, 5], [5, 5, 5]],
[[6, 6], [6, 6]]
]
# 方块颜色
COLORS = [
(0, 0, 0),
(255, 0, 0),
(0, 255, 0),
(0, 0, 255),
(255, 255, 0),
(0, 255, 255),
(255, 0, 255)
]
# 初始化 Pygame 库
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
# 设置窗口标题
pygame.display.set_caption('俄罗斯方块')
# 创建字体对象
font = pygame.font.Font(None, 36)
# 计时器
clock = pygame.time.Clock()
# 方块类
class Block:
def __init__(self):
self.x = SCREEN_WIDTH // 2 // BLOCK_SIZE * BLOCK_SIZE
self.y = 0
self.type = random.randint(0, len(BLOCKS) - 1)
self.rotation = 0
self.color = COLORS[self.type + 1]
self.shape = BLOCKS[self.type][self.rotation]
# 检查方块是否碰到边界或其他方块
def check_collision(self, x, y, shape):
for i in range(len(shape)):
for j in range(len(shape[i])):
if shape[i][j] != 0:
if y + i * BLOCK_SIZE >= SCREEN_HEIGHT:
return True
if x + j * BLOCK_SIZE < 0 or x + j * BLOCK_SIZE >= SCREEN_WIDTH:
return True
if board[(y + i * BLOCK_SIZE) // BLOCK_SIZE][(x + j * BLOCK_SIZE) // BLOCK_SIZE] != 0:
return True
return False
# 旋转方块
def rotate(self):
new_rotation = (self.rotation + 1) % len(BLOCKS[self.type])
new_shape = BLOCKS[self.type][new_rotation]
if not self.check_collision(self.x, self.y, new_shape):
self.rotation = new_rotation
self.shape = new_shape
# 移动方块
def move(self, dx, dy):
if not self.check_collision(self.x + dx, self.y + dy, self.shape):
self.x += dx
self.y += dy
# 加入方块到游戏区域
def join(self):
for i in range(len(self.shape)):
for j in range(len(self.shape[i])):
if self.shape[i][j] != 0:
board[(self.y + i * BLOCK_SIZE) // BLOCK_SIZE][(self.x + j * BLOCK_SIZE) // BLOCK_SIZE] = self.type + 1
# 更新方块状态
def update(self):
if not self.check_collision(self.x, self.y + BLOCK_SIZE, self.shape):
self.y += BLOCK_SIZE
else:
self.join()
self.__init__()
# 初始化游戏区域
board = [[0] * (SCREEN_WIDTH // BLOCK_SIZE) for _ in range(SCREEN_HEIGHT // BLOCK_SIZE)]
# 当前方块
current_block = Block()
# 游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
current_block.move(-BLOCK_SIZE, 0)
elif event.key == pygame.K_RIGHT:
current_block.move(BLOCK_SIZE, 0)
elif event.key == pygame.K_UP:
current_block.rotate()
elif event.key == pygame.K_DOWN:
current_block.move(0, BLOCK_SIZE)
# 更新游戏状态
current_block.update()
# 绘制游戏区域
screen.fill((255, 255, 255))
for i in range(len(board)):
for j in range(len(board[i])):
if board[i][j] != 0:
pygame.draw.rect(screen, COLORS[board[i][j]], (j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
for i in range(len(current_block.shape)):
for j in range(len(current_block.shape[i])):
if current_block.shape[i][j] != 0:
pygame.draw.rect(screen, current_block.color, (current_block.x + j * BLOCK_SIZE, current_block.y + i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE))
# 绘制得分
score = sum([1 for row in board if all(row)])
text = font.render('Score: {}'.format(score), True, (0, 0, 0))
screen.blit(text, (10, 10))
# 更新屏幕
pygame.display.update()
# 设置帧率
clock.tick(10)
```
运行该代码,即可在 Pygame 窗口中玩俄罗斯方块游戏。通过方向键左右移动方块,上键旋转方块,下键加速下落,得分规则为消除一行得一分。
阅读全文
相关推荐
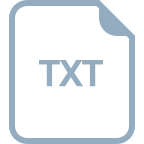
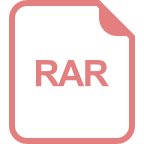
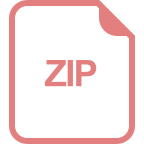


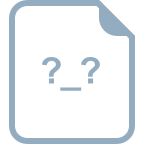
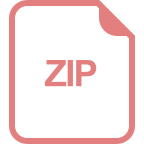
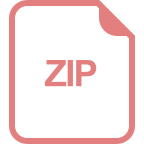
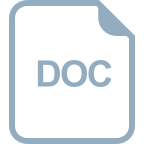







