建立链式多项式类,并设计算法实现多项式的相加。(c++编写)
时间: 2024-11-04 21:11:28 浏览: 23
在C++中,我们可以创建一个链表结构表示多项式,每个节点包含系数和下一个节点的引用。以下是一个简单的链式多项式类的实现,以及用于两个多项式相加的算法:
```cpp
#include <iostream>
using namespace std;
// 定义链表节点
struct Term {
double coefficient;
int exponent;
Term* next;
};
// 链式多项式类
class Polynomial {
private:
Term* head;
public:
Polynomial() : head(nullptr) {}
// 添加项到多项式
void addTerm(double c, int e) {
Term* newNode = new Term{c, e, nullptr};
if (head == nullptr) {
head = newNode;
} else {
Term* current = head;
while (current->next != nullptr && current->exponent < e) {
current = current->next;
}
if (current->exponent == e) {
current->coefficient += c;
} else {
newNode->next = current->next;
current->next = newNode;
}
}
}
// 打印多项式
void print() {
Term* temp = head;
while (temp != nullptr) {
cout << temp->coefficient << "x^" << temp->exponent << " + ";
temp = temp->next;
}
cout << "\n";
}
// 相加操作
Polynomial operator+(const Polynomial& other) const {
Polynomial result(*this);
Term* curr = head;
Term* otherCurr = other.head;
while (curr != nullptr && otherCurr != nullptr) {
if (curr->exponent > otherCurr->exponent) {
curr = curr->next;
} else if (curr->exponent < otherCurr->exponent) {
otherCurr = otherCurr->next;
} else {
result.addTerm(curr->coefficient + otherCurr->coefficient, curr->exponent);
curr = curr->next;
otherCurr = otherCurr->next;
}
}
// 将剩余项添加到结果
while (curr != nullptr) {
result.addTerm(curr->coefficient, curr->exponent);
curr = curr->next;
}
while (otherCurr != nullptr) {
result.addTerm(otherCurr->coefficient, otherCurr->exponent);
otherCurr = otherCurr->next;
}
return result;
}
};
int main() {
Polynomial p1;
p1.addTerm(2, 0); // 2x^0
p1.addTerm(3, 1); // 3x^1
Polynomial p2;
p2.addTerm(4, 0); // 4x^0
p2.addTerm(-1, 2); // -1x^2
Polynomial sum = p1 + p2;
sum.print(); // 输出: 5x^0 + 2x^1 - x^2 +
return 0;
}
```
在这个例子中,`Polynomial` 类实现了多项式的存储和打印功能,`operator+` 运算符则实现了两个多项式的相加。在 `main` 函数中,我们创建了两个多项式并求和。
阅读全文
相关推荐
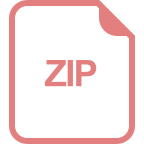
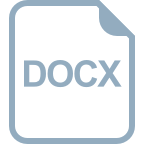
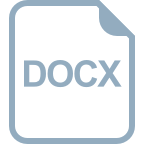
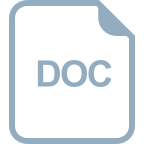
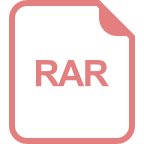
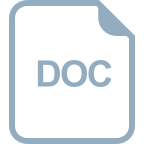
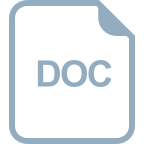
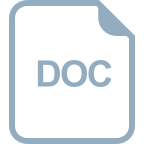
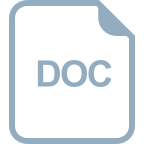
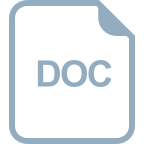
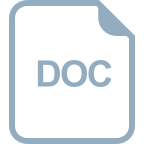
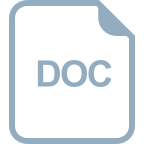






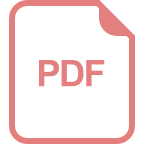