写一个俄罗斯方块的代码
时间: 2023-12-01 18:13:07 浏览: 33
以下是一个简单的俄罗斯方块游戏的代码示例,使用 Python 和 Pygame 库实现:
```python
import pygame
import random
# 初始化 Pygame 库
pygame.init()
# 定义游戏区域的大小
WIDTH = 800
HEIGHT = 600
# 定义每个小方块的大小
BLOCK_SIZE = 30
# 定义游戏区域的行列数
ROWS = HEIGHT // BLOCK_SIZE
COLS = WIDTH // BLOCK_SIZE
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义方块类型
SQUARES = [
[[1, 1], [1, 1]],
[[0, 2, 0], [2, 2, 2]],
[[0, 3, 3], [3, 3, 0]],
[[4, 4, 0], [0, 4, 4]],
[[0, 5, 0, 0], [0, 5, 5, 5]],
[[0, 0, 6], [6, 6, 6], [0, 0, 0]],
[[7, 7, 0], [0, 7, 7], [0, 0, 0]],
]
# 定义方块类
class Block:
def __init__(self, x, y, shape):
self.x = x
self.y = y
self.shape = shape
def draw(self, surface):
for row in range(len(self.shape)):
for col in range(len(self.shape[row])):
if self.shape[row][col] != 0:
pygame.draw.rect(
surface,
WHITE,
(
(self.x + col) * BLOCK_SIZE,
(self.y + row) * BLOCK_SIZE,
BLOCK_SIZE,
BLOCK_SIZE,
),
)
def move(self, dx, dy):
self.x += dx
self.y += dy
def rotate(self):
self.shape = [[self.shape[j][i] for j in range(len(self.shape))] for i in range(len(self.shape[0]) - 1, -1, -1)]
# 定义游戏类
class Game:
def __init__(self):
self.screen = pygame.display.set_mode((WIDTH, HEIGHT))
self.clock = pygame.time.Clock()
self.score = 0
self.board = [[0] * COLS for _ in range(ROWS)]
self.block = self.new_block()
def run(self):
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
self.block.move(-1, 0)
elif event.key == pygame.K_RIGHT:
self.block.move(1, 0)
elif event.key == pygame.K_DOWN:
self.block.move(0, 1)
elif event.key == pygame.K_UP:
self.block.rotate()
# 更新游戏状态
if self.is_valid_move(0, 1):
self.block.move(0, 1)
else:
self.freeze_block()
self.clear_lines()
self.block = self.new_block()
# 绘制游戏界面
self.screen.fill(BLACK)
self.draw_board()
self.block.draw(self.screen)
pygame.display.update()
# 控制游戏帧率
self.clock.tick(10)
def new_block(self):
return Block(COLS // 2, 0, random.choice(SQUARES))
def is_valid_move(self, dx, dy):
for row in range(len(self.block.shape)):
for col in range(len(self.block.shape[row])):
if self.block.shape[row][col] != 0:
x = self.block.x + col + dx
y = self.block.y + row + dy
if x < 0 or x >= COLS or y >= ROWS or self.board[y][x] != 0:
return False
return True
def freeze_block(self):
for row in range(len(self.block.shape)):
for col in range(len(self.block.shape[row])):
if self.block.shape[row][col] != 0:
x = self.block.x + col
y = self.block.y + row
self.board[y][x] = self.block.shape[row][col]
self.block = None
def clear_lines(self):
lines_cleared = 0
for row in range(ROWS):
if all(self.board[row]):
del self.board[row]
self.board.insert(0, [0] * COLS)
lines_cleared += 1
self.score += lines_cleared ** 2
def draw_board(self):
for row in range(ROWS):
for col in range(COLS):
if self.board[row][col] != 0:
pygame.draw.rect(
self.screen,
WHITE,
(
col * BLOCK_SIZE,
row * BLOCK_SIZE,
BLOCK_SIZE,
BLOCK_SIZE,
),
)
# 运行游戏
if __name__ == "__main__":
game = Game()
game.run()
```
这个代码示例实现了一个基本的俄罗斯方块游戏,包括方块的移动、旋转和下落,方块的冻结和消除行,分数计算等基本功能。可以通过修改方块类型、颜色、游戏区域大小等参数来自定义游戏。
相关推荐
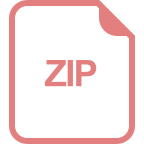












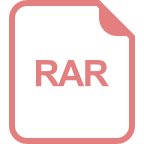
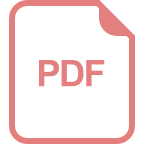