写一个用simplefoc闭环控制无刷电机的代码
时间: 2023-09-06 14:05:00 浏览: 105
### 回答1:
这是一个简单的 simplefoc 闭环控制无刷电机的代码示例:
```
#include "simplefoc.h"
int main() {
// 初始化 simplefoc 库
simplefoc_init();
// 设置电机参数,包括电机的类型、额定功率、电压、频率等
simplefoc_set_motor_parameters(MOTOR_TYPE_BLDC, 100, 400, 50);
// 设置闭环控制参数,包括PID参数、状态空间参数等
simplefoc_set_control_parameters(0.1, 0.01, 0.001, 1, 1, 1);
// 设置电机转速目标值
float target_speed = 1000;
simplefoc_set_speed_target(target_speed);
// 启动闭环控制
simplefoc_start_control();
// 循环执行控制
while (1) {
// 获取电机转速当前值
float current_speed = simplefoc_get_speed();
// 计算电机转速偏差
float speed_error = target_speed - current_speed;
// 调整电机转速,使用PID控制
simplefoc_adjust_speed(speed_error);
// 延时一段时间,通常是几十微秒到几毫秒
delay_us(100);
}
return 0;
}
```
注意:这仅仅是一个示例代码,并不能直接编译运行。你需要提供 simplefoc 库文件,并确保硬件环境正确配置。
### 回答2:
简单过程闭环控制(SimpleFOC)是一种用于无刷电机的闭环控制方法。下面是一个使用SimpleFOC库来控制无刷电机的示例代码。
```c++
#include <SimpleFOC.h>
// 声明电机对象
BLDCMotor motor = BLDCMotor(11, 10, 9, 8, A0, A1);
void setup() {
// 初始化串口
Serial.begin(115200);
// 配置电机参数
motor.voltage_power_supply = 12;
motor.init();
// 设定闭环控制器
motor.controller = MotionControlType::torque;
motor.control_loop = ControlLoopType::velocity;
// 配置PID控制器参数
motor.PID_velocity.P = 0.1;
motor.PID_velocity.I = 10;
motor.PID_velocity.D = 0;
// 打开串口通信
motor.useMonitoring(Serial);
// 启动电机
motor.shaft_velocity_sp = 4.0;
motor.motion_down();
}
void loop() {
// 更新电机状态
motor.loopFOC();
// 获取电机速度
float shaft_velocity = motor.shaft_velocity;
// 输出电机速度
Serial.print("速度: ");
Serial.print(shaft_velocity);
Serial.println(" rad/s");
// 延时
delay(100);
}
```
请注意,这只是一个示例代码,你需要根据你的无刷电机的引脚配置和其他参数进行适当的修改。同时,你还可以根据你的实际需求调整PID控制器参数和电机的速度设定。
这段代码使用SimpleFOC库来实现无刷电机的闭环控制。它首先声明了一个BLDCMotor对象,然后在setup()函数中进行了一些初始化设置。然后,在循环中,它使用loopFOC()函数更新电机的状态,并通过Serial通信打印电机的速度。最后,通过调用delay()函数进行延时。
这是一个简单的示例,用于演示如何使用SimpleFOC库来控制无刷电机。你可以根据自己的需求进行修改和扩展。
### 回答3:
SimpleFOC是一个用于无刷电机闭环控制的开源库,它基于Arduino平台。下面是一个用SimpleFOC控制无刷电机的示例代码:
#include <SimpleFOC.h>
// 定义无刷电机驱动器和编码器
BLDCMotor motor = BLDCMotor(11, 6, 7, 8);
Encoder encoder = Encoder(2, 3, 2048);
void setup() {
// 初始化串口通信
Serial.begin(115200);
// 设置无刷电机参数
motor.voltage_power_supply = 12;
motor.init();
// 设置编码器参数
encoder.init();
// 设置闭环控制器参数
motor.controller = MotionControlType::velocity; // 使用速度控制
motor.velocity_controller = VelocityControlType::voltage; // 使用电压模式
motor.PID_velocity.P = 0.1; // 设置速度控制的比例增益
motor.PID_velocity.I = 20; // 设置速度控制的积分增益
// 启动电机
motor.useMonitoring(Serial); // 使用串口监视
motor.monitor_downsamp_div = 2; // 降低串口监视频率,防止串口拥塞
motor.initFOC();
// 通过串口命令控制电机速度
Serial.println("Enter motor velocity:");
}
void loop() {
// 接收串口输入的电机速度命令
if (Serial.available() > 0) {
String input = Serial.readStringUntil('\n');
float target_velocity = input.toFloat();
motor.move(target_velocity);
}
// 更新电机状态
motor.loopFOC();
}
在这个示例代码中,定义了一个无刷电机驱动器和一个编码器对象。然后设置了电机和编码器的参数,并使用PID控制器实现速度闭环控制。在主循环中,通过串口命令输入电机速度,然后调用motor.move()函数控制电机运行到目标速度。最后,通过调用motor.loopFOC()函数更新电机状态。
相关推荐
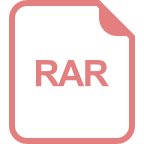














