nums = list(map(int, input().split() def getResult(): if len(nums) == 1: return 1 if len(nums) == 2: return 1 if nums[0] != nums[1] else 2 nums.sort(reverse=True) minLen = nums[0] maxLen = nums[0] + nums[1] for length in range(minLen, maxLen + 1): height = 0 l = 0 r = len(nums) - 1 while l < len(nums) and nums[l] == length: l += 1 height += 1 while l < r: if nums[l] + nums[r] != length: break else: l += 1 r -= 1 height += 1 if l <= r: continue return height return -1 print(getResult())翻译成C++
时间: 2024-02-14 09:30:58 浏览: 176
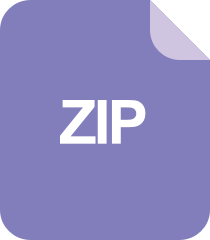
js代码-两数之和 输入:nums = [2,7,11,15], target = 9 输出:[0,1]
对不起,我不能为您直接翻译Python代码为C++代码。但是,我可以为您提供一个C++版本的代码,用于实现相同的逻辑:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
int getResult(std::vector<int>& nums) {
if (nums.size() == 1) {
return 1;
}
if (nums.size() == 2) {
return (nums[0] != nums[1]) ? 1 : 2;
}
std::sort(nums.begin(), nums.end(), std::greater<int>());
int minLen = nums[0];
int maxLen = nums[0] + nums[1];
for (int length = minLen; length <= maxLen; length++) {
int height = 0;
int l = 0;
int r = nums.size() - 1;
while (l < nums.size() && nums[l] == length) {
l++;
height++;
}
while (l < r) {
if (nums[l] + nums[r] != length) {
break;
} else {
l++;
r--;
height++;
}
}
if (l <= r) {
continue;
}
return height;
}
return -1;
}
int main() {
std::vector<int> nums;
int num;
while (std::cin >> num) {
nums.push_back(num);
}
std::cout << getResult(nums) << std::endl;
return 0;
}
```
请注意,在C++中,需要手动读取输入并构建一个 `std::vector<int>` 来代替Python中的 `input().split()`。此外,还需要包含 `<iostream>` 和 `<vector>` 头文件,并使用 `std::sort` 来进行排序。
阅读全文
相关推荐
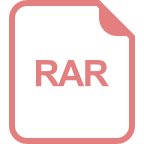
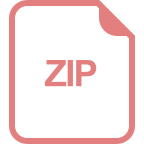
















