通过python实现对频域模糊处理图像的复原
时间: 2023-06-08 18:08:49 浏览: 63
好的,您需要通过Python实现频域模糊处理图像的复原。这个问题需要使用频域滤波来处理图像。以下是实现这个过程的一些步骤:
1. 读取输入图像和模糊核图像
2. 对输入图像和模糊核进行傅里叶变换
3. 将傅里叶变换后的模糊核图像除以傅里叶变换后的输入图像
4. 对傅里叶变换后的输入图像和除法结果进行点乘
5. 对点乘结果进行逆傅里叶变换
这些步骤将复原您的图像。请注意,这个问题的实现可能需要使用Python中的一些库,如numpy,cv2和matplotlib等。
相关问题
通过python实现对彩色图像的频域模糊及复原
可以通过将彩色图像转换为RGB三个通道的灰度图像,然后分别进行频域模糊和复原。
1. 首先,使用OpenCV库将彩色图像读入,并将其转换为灰度图像:
```python
import cv2
img = cv2.imread('color_image.jpg')
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 然后,使用numpy库中的fft2函数将灰度图像转换为频域图像,并进行频域滤波,例如低通滤波器:
```python
import numpy as np
# 2D fast Fourier transform to convert image to frequency domain
f = np.fft.fft2(gray_img)
# Shift the zero-frequency component to the center of the spectrum
fshift = np.fft.fftshift(f)
# Create a low pass filter to remove high frequency components
rows, cols = gray_img.shape
crow, ccol = rows//2, cols//2
mask = np.zeros((rows, cols), np.uint8)
mask[crow-30:crow+30, ccol-30:ccol+30] = 1
# Apply the mask to the frequency domain image
fshift = fshift * mask
# Shift the zero-frequency component back to the corners of the spectrum
f_ishift = np.fft.ifftshift(fshift)
# 2D inverse fast Fourier transform to convert frequency domain image to spatial image
img_back = np.fft.ifft2(f_ishift)
# Convert the real part of the complex image to an 8-bit grayscale image
img_back = np.abs(img_back).astype(np.uint8)
```
3. 最后,显示频域模糊和复原图像:
```python
# Show the original image, frequency domain blurred image, and recovered image
cv2.imshow('Original Image', img)
cv2.imshow('Frequency Domain Blurred Image', img_back)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这样就可以通过Python实现对彩色图像的频域模糊及复原了。
通过python实现对彩色图像的频域模糊及复原,在频域模糊前进行相应预处理,注释用中文显示
很高兴能与您进行对话,我明白您的问题并将为您提供帮助。
对于彩色图像的频域模糊和复原,您可以使用Python和OpenCV库来实现。下面是一些简要的步骤:
1. 导入所需的库和图像:您需要安装并导入OpenCV库,并加载图像到Python中,如下所示:
```python
import cv2
import numpy as np
# 加载彩色图像
img = cv2.imread('color_image.jpg')
```
2. 颜色空间转换:在进行频域模糊之前,您需要将图像从RGB颜色空间转换为灰度或HSV颜色空间。这是因为频域模糊只在灰度或单个通道颜色下进行。您可以使用以下代码完成颜色空间转换:
```python
#将图像转换为HSV颜色空间
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
#或将图像转换为灰度
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
3. 进行频域模糊:在Python中,通常使用傅里叶变换(FFT)对图像进行频域转换。您可以使用以下代码使用FFT对图像进行频域转换和模糊:
```python
# 进行傅里叶变换
f = np.fft.fft2(gray)
# 将FFT数据移动到中心
fshift = np.fft.fftshift(f)
# 创建一个掩码,以便在中心创建2个同心圆
rows, cols = gray.shape
crow, ccol = int(rows / 2), int(cols / 2)
mask = np.zeros((rows, cols), np.uint8)
mask = cv2.circle(mask, (ccol, crow), 50, (1, 1, 1), -1)
mask = cv2.circle(mask, (ccol, crow), 20, (0, 0, 0), -1)
# 应用掩码并进行逆变换
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
```
在上述代码中,您可以使用掩码创建2个同心圆来模拟模糊效果。调整同心圆的半径可以模拟模糊程度。在应用掩码之后,您需要进行逆傅里叶变换以将图像转换回时域。
4. 进行图像复原:经过频域模糊后,您可以使用以下代码对图像进行复原:
```python
# 进行傅里叶变换
f = np.fft.fft2(img_back)
# 将FFT数据移动到中心
fshift = np.fft.fftshift(f)
# 应用逆掩码并进行逆变换
fshift = fshift / mask
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
```
在上述代码中,您需要使用逆掩码来还原原始图像。然后,您需要进行逆傅里叶变换以将图像转换回时域。
以上是一些简要的步骤,您可以根据您的需求进行相应的修改和调整。希望这些信息对您有所帮助!
相关推荐
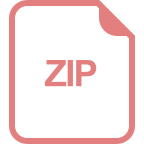
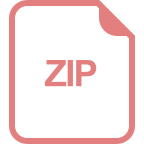












