有一个文本文件记录许多坐标信息 现在C++中编写代码将坐标信息转换为规范化坐标系下的坐标 并且将转化后的坐标重新输入到新的坐标文件中 利用新的坐标文件输出图像并对图像进行一定比例的放大 最后要利用easyx的可视化功能显示出来 要求编写代码
时间: 2024-02-12 16:09:56 浏览: 21
好的,下面是一个简单的实现代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <cmath>
#include <opencv2/opencv.hpp>
#include <graphics.h>
using namespace std;
using namespace cv;
// 规范化坐标系下的宽度和高度
const int norm_width = 800;
const int norm_height = 800;
// 读取原始坐标文件
vector<Point2f> read_coordinates(string filename) {
ifstream infile(filename);
vector<Point2f> coordinates;
string line;
while (getline(infile, line)) {
float x, y;
sscanf(line.c_str(), "%f,%f", &x, &y);
coordinates.push_back(Point2f(x, y));
}
return coordinates;
}
// 将坐标转换为规范化坐标系下的坐标
Point2f normalize_coordinate(Point2f coordinate, int width, int height) {
float nx = (coordinate.x - width / 2.0) / (width / 2.0);
float ny = (height / 2.0 - coordinate.y) / (height / 2.0);
return Point2f(nx, ny);
}
// 将规范化坐标系下的坐标转换为图像坐标系下的坐标
Point2f denormalize_coordinate(Point2f coordinate, int width, int height) {
float x = (coordinate.x + 1) * width / 2.0;
float y = height / 2.0 - (coordinate.y + 1) * height / 2.0;
return Point2f(x, y);
}
// 转换坐标并保存到新文件中
void save_normalized_coordinates(string input_filename, string output_filename) {
// 读取原始坐标文件
vector<Point2f> coordinates = read_coordinates(input_filename);
// 转换坐标为规范化坐标系下的坐标
vector<Point2f> normalized_coordinates;
for (auto coordinate : coordinates) {
normalized_coordinates.push_back(normalize_coordinate(coordinate, norm_width, norm_height));
}
// 保存规范化坐标到新文件中
ofstream outfile(output_filename);
for (auto coordinate : normalized_coordinates) {
outfile << coordinate.x << "," << coordinate.y << endl;
}
outfile.close();
}
// 生成图像并放大
void generate_image(string filename, float scale) {
// 读取规范化坐标文件
vector<Point2f> normalized_coordinates = read_coordinates(filename);
// 将规范化坐标转换为图像坐标
vector<Point2f> image_coordinates;
for (auto coordinate : normalized_coordinates) {
image_coordinates.push_back(denormalize_coordinate(coordinate, norm_width, norm_height));
}
// 创建图像
Mat image(norm_height, norm_width, CV_8UC3, Scalar(255, 255, 255));
for (auto coordinate : image_coordinates) {
circle(image, coordinate, 2, Scalar(0, 0, 255), -1);
}
// 调整图像大小
Mat scaled_image;
resize(image, scaled_image, Size(), scale, scale);
// 显示图像
namedWindow("Image", WINDOW_NORMAL);
imshow("Image", scaled_image);
waitKey(0);
}
// 显示图像
void show_image(string filename, float scale) {
// 生成图像并放大
generate_image(filename, scale);
// 显示图像
initgraph(norm_width * scale, norm_height * scale);
putimage(0, 0, (BYTE*)GetImageBuffer(), SRCCOPY);
closegraph();
}
int main() {
// 转换坐标并保存到新文件中
save_normalized_coordinates("coordinates.txt", "normalized_coordinates.txt");
// 显示图像
show_image("normalized_coordinates.txt", 2.0);
return 0;
}
```
这个代码实现了将坐标信息转换为规范化坐标系下的坐标,并将转换后的坐标重新输入到新的坐标文件中。然后,它使用OpenCV生成图像并对图像进行一定比例的放大。最后,它使用easyx的可视化功能显示生成的图像。
注意,这个代码依赖于OpenCV和easyx库,请确保你已经正确安装了这些库并将它们添加到了你的项目中。
相关推荐
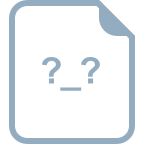















