遗传算法函数求最大值python实现
时间: 2024-12-02 12:12:18 浏览: 20
遗传算法是一种模拟自然选择和遗传过程的优化搜索技术,常用于解决复杂函数的全局优化问题。在Python中,我们可以使用`deap`库来实现遗传算法,下面是一个简单的例子,展示如何使用它来寻找一个函数的最大值:
```python
from deap import base, creator, tools
# 定义问题域、适应度函数和个体编码
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
def eval_one_max(individual):
return -sum(individual), # 这里假设我们是在找最小值,所以需要取负值
toolbox = base.Toolbox()
toolbox.register("attr_bool", random.randint, 0, 1) # 使用二进制编码,1代表True,0代表False
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_bool, n=len(individual)) # 初始化个体
toolbox.register("population", tools.initRepeat, list, toolbox.individual) # 初始化种群
toolbox.register("evaluate", eval_one_max)
toolbox.register("mate", tools.cxTwoPoint) # 交叉操作
toolbox.register("mutate", tools.mutFlipBit, indpb=0.1) # 突变操作
toolbox.register("select", tools.selTournament, tournsize=3) # 选择操作
def main(pop_size, generations):
pop = toolbox.population(n=pop_size)
hof = tools.HallOfFame(1) # 保存最佳解
stats = tools.Statistics(lambda ind: ind.fitness.values[0]) # 记录适应度
stats.register("avg", numpy.mean)
stats.register("std", numpy.std)
stats.register("min", numpy.min)
stats.register("max", numpy.max)
for gen in range(generations):
offspring = tools.select(pop, len(pop))
offspring = [toolbox.clone(ind) for ind in offspring]
# Crossover and mutation
offspring = toolbox.mate(offspring)
offspring = [toolbox.clone(ind) for ind in offspring]
toolbox.mutate(offspring)
del offspring[:len(pop)] # Replace the worst individuals with new ones
# Evaluate the fitness of the offspring
fitnesses = map(toolbox.evaluate, offspring)
for ind, fit in zip(offspring, fitnesses):
ind.fitness.values = fit
# Update statistics
stats.update(offspring)
# Select the next generation population
pop[:] = offspring
# Save the best solution found so far
hof.update(offspring)
return hof[0], stats
best_solution, stats = main(population_size=50, generations=100)
print(f"Best solution: {best_solution}")
print(f"Statistics: {stats}")
```
这个脚本会尝试在一个给定的迭代次数(`generations`)内找到一个函数的最大值,通过不断的进化和选择操作。请注意,这个例子假设了`eval_one_max`是你想要优化的具体函数,你需要替换为你实际的目标函数。
阅读全文
相关推荐
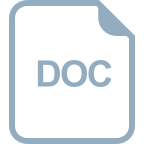
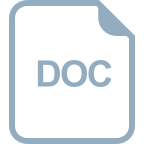
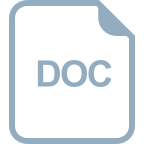








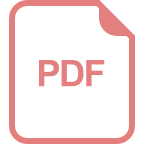






