利用C++编写一个学生类,学生信息包括姓名、学号、年龄、性别和成绩;统计学生的总人及总成绩,并输出。
时间: 2024-10-12 21:16:18 浏览: 32
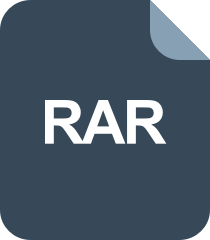
设计一个学生类Student(学生学号、姓名、数学、英语、计算机成绩;)

在C++中,你可以创建一个名为`Student`的类来表示学生的信息,然后定义一些成员变量以及相应的成员函数来实现所需的功能。以下是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
class Student {
public:
// 构造函数
Student(std::string name, int id, int age, char gender, double score)
: name(name), student_id(id), age(age), gender(gender), score(score) {}
// 获取学生信息
std::string getName() const { return name; }
int getStudentId() const { return student_id; }
int getAge() const { return age; }
char getGender() const { return gender; }
double getScore() const { return score; }
// 输出学生信息
void displayInfo() const {
std::cout << "Name: " << name << ", ID: " << student_id << ", Age: " << age
<< ", Gender: " << gender << ", Score: " << score << std::endl;
}
private:
std::string name;
int student_id;
int age;
char gender;
double score;
};
int main() {
// 创建一个学生列表
std::vector<Student> students = {
{"Alice", 1001, 18, 'F', 90.5},
{"Bob", 1002, 17, 'M', 88.0},
{"Charlie", 1003, 19, 'M', 92.0}
};
// 统计学生总数
int total_students = students.size();
std::cout << "Total students: " << total_students << std::endl;
// 计算并输出总成绩
double total_score = 0.0;
for (const auto& student : students) {
total_score += student.getScore();
}
std::cout << "Total score: " << total_score << std::endl;
// 遍历学生列表并显示每个学生的详细信息
for (const auto& student : students) {
student.displayInfo();
}
return 0;
}
```
在这个例子中,我们首先定义了一个`Student`类,包含名字、学号、年龄、性别和成绩等属性,还提供了一些获取和显示信息的公共方法。在`main`函数中,我们创建了一个学生向量,统计了学生数量和总成绩,并打印出每个学生的详细信息。
阅读全文
相关推荐
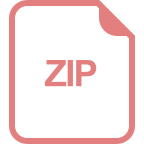
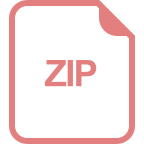














