设计一个表示形状的类shape,以及从shape继承的表示矩形的类rectangle和表示椭圆的类ellipse。shape类用一个表示坐标点的类point的对象来表达它的左上角坐标,用一个表示尺寸的类dimension的对象来表示它的大小。 你的程序要严格按照所给的类和函数的声明来实现。
时间: 2023-05-31 18:18:12 浏览: 127
### 回答1:
好的,下面是用中文回复:
题目要求设计一个表示形状的类shape,以及从shape继承的表示矩形的类rectangle和表示椭圆的类ellipse。其中,shape类用一个表示坐标点的类point的对象来表达它的左上角坐标,用一个表示尺寸的类dimension的对象来表示它的大小。
具体实现时,需要按照所给的类和函数的声明来编写程序。可以先定义point类和dimension类,再定义shape类,并在其中包含point和dimension对象。接着,分别定义rectangle类和ellipse类,让它们继承shape类,并在其中实现相应的函数。
需要注意的是,程序要严格按照题目要求来实现,确保类和函数的声明与题目一致。同时,还需要考虑代码的可读性和可维护性,尽量使用清晰简洁的代码来实现功能。
### 回答2:
设计一个表示形状的类shape,以及从shape继承的表示矩形的类rectangle和表示椭圆的类ellipse。
首先,我们需要定义point类,它包含两个成员变量,分别表示横坐标和纵坐标。然后,我们定义dimension类,它包含两个成员变量,分别表示宽度和高度。最后,我们定义shape类,它包含两个成员变量,分别表示左上角的坐标和尺寸大小。
class point {
public:
int x, y;
point(int a = 0, int b = 0) { x = a; y = b; }
};
class dimension {
public:
int width, height;
dimension(int w = 0, int h = 0) { width = w; height = h; }
};
class shape {
public:
point p;
dimension d;
shape(point a, dimension b) { p = a; d = b; }
};
现在,我们可以定义一个表示矩形的类rectangle,该类从shape类中继承。它包含一个成员函数,用于计算矩形的面积。该函数使用shape类中的成员变量来计算矩形的面积,并将结果返回。
class rectangle : public shape {
public:
rectangle(point a, dimension b) : shape(a, b) {}
int area() { return d.width * d.height; }
};
同样地,我们定义一个表示椭圆的类ellipse,该类从shape类中继承。它包含一个成员函数,用于计算椭圆的面积。该函数使用shape类中的成员变量来计算椭圆的面积,并将结果返回。
class ellipse : public shape {
public:
ellipse(point a, dimension b) : shape(a, b) {}
int area() { return 3.14 * d.width * d.height; }
};
现在,我们可以使用这些类来创建各种形状。例如,我们可以创建一个矩形对象,通过调用它的area()函数来计算面积。同样地,我们也可以创建一个椭圆对象,并通过它的area()函数来计算面积。
int main() {
point p1(0, 0);
dimension d1(2, 4);
rectangle r1(p1, d1);
cout << "Rectangle area: " << r1.area() << endl;
point p2(0, 0);
dimension d2(2, 4);
ellipse e1(p2, d2);
cout << "Ellipse area: " << e1.area() << endl;
return 0;
}
总之,上述代码演示了如何定义一个表示形状的类shape,并从中继承表示矩形的类rectangle和表示椭圆的类ellipse。我们可以在程序中创建各种形状的对象,并调用它们的成员函数来计算面积。这个示例代码提供了一种用C++实现形状类层次结构的方法。
### 回答3:
设计一个表示形状的类Shape,作为基类,包含左上角点的坐标和尺寸两个数据成员,以及相应的构造函数、拷贝构造函数和虚析构函数。为了实现这个类,我们需要一个表示坐标点的类Point和一个表示尺寸的类Dimension。
class Point{
private:
int x, y;
public:
Point(int _x=0, int _y=0): x(_x), y(_y){}
int get_x() const{return x;}
int get_y() const{return y;}
};
class Dimension{
private:
int width, height;
public:
Dimension(int _width=0, int _height=0): width(_width), height(_height){}
int get_width() const{return width;}
int get_height() const{return height;}
};
class Shape{
private:
Point position;
Dimension size;
public:
Shape(Point _position, Dimension _size): position(_position), size(_size){}
Shape(const Shape& other): position(other.position), size(other.size){}
virtual ~Shape(){}
Point get_position() const{return position;}
Dimension get_size() const{return size;}
};
接下来,我们需要用继承的方式派生出表示矩形的类Rectangle和表示椭圆的类Ellipse。因为矩形和椭圆的形状不同,所以我们需要在派生类中实现它们的特殊构造函数和虚析构函数。
class Rectangle: public Shape{
public:
Rectangle(Point _position, Dimension _size): Shape(_position, _size){}
Rectangle(const Rectangle& other): Shape(other){}
virtual ~Rectangle(){}
};
class Ellipse: public Shape{
public:
Ellipse(Point _position, Dimension _size): Shape(_position, _size){}
Ellipse(const Ellipse& other): Shape(other){}
virtual ~Ellipse(){}
};
以上就是一个简单的面向对象的设计实现,通过使用继承方式派生出更具体的类来描述不同形状。Shape类包含共性成员数据和方法,Rectangle和Ellipse类则分别具有自己的数据和特性。这样的设计不仅能够提高代码的可维护性和可扩展性,还可以提供代码的重用性,避免重复的代码编写。
相关推荐
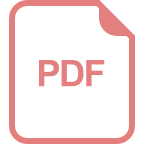
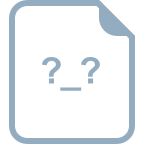
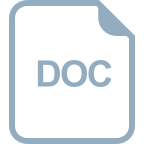














