在屏幕上绘制一个三角形,指定三个顶点的颜色分别为红、绿、蓝三色。用边标志填充算法光滑着色填充该三角形。
时间: 2024-05-03 14:19:44 浏览: 18
这是一个比较底层的图形学问题,需要使用特定的图形库或者编写自己的算法来实现。以下是使用Python和Pygame库实现的代码示例:
```python
import pygame
# 初始化Pygame
pygame.init()
# 设置窗口大小和标题
win_width = 640
win_height = 480
win = pygame.display.set_mode((win_width, win_height))
pygame.display.set_caption("Smooth Color Triangle")
# 定义三个顶点的坐标和颜色
vertices = [(100, 100, (255, 0, 0)), (300, 100, (0, 255, 0)), (200, 300, (0, 0, 255))]
# 计算三角形的边界框
xmin = min(vertices, key=lambda v: v[0])[0]
xmax = max(vertices, key=lambda v: v[0])[0]
ymin = min(vertices, key=lambda v: v[1])[1]
ymax = max(vertices, key=lambda v: v[1])[1]
# 定义边界框内每个像素点的颜色
colors = []
for y in range(ymin, ymax+1):
for x in range(xmin, xmax+1):
if (x, y) in [(v[0], v[1]) for v in vertices]:
# 如果该点是三角形的顶点,则直接使用该顶点的颜色
color = [v[2] for v in vertices if (v[0], v[1]) == (x, y)][0]
else:
# 否则,计算该点在三角形内的重心坐标
a = (vertices[1][1] - vertices[2][1])*(x - vertices[2][0]) + (vertices[2][0] - vertices[1][0])*(y - vertices[2][1])
b = (vertices[2][1] - vertices[0][1])*(x - vertices[2][0]) + (vertices[0][0] - vertices[2][0])*(y - vertices[2][1])
c = (vertices[0][1] - vertices[1][1])*(x - vertices[0][0]) + (vertices[1][0] - vertices[0][0])*(y - vertices[0][1])
alpha = a / (a + b + c)
beta = b / (a + b + c)
gamma = c / (a + b + c)
# 根据重心坐标计算该点的颜色
color = tuple(int(alpha*v[2][0] + beta*v[2][1] + gamma*v[2][2]) for v in vertices)
colors.append(color)
# 在窗口中绘制边界框内的像素点
for y in range(ymin, ymax+1):
for x in range(xmin, xmax+1):
index = (y - ymin)*(xmax - xmin + 1) + (x - xmin)
pygame.draw.rect(win, colors[index], (x, y, 1, 1))
# 更新窗口并等待退出事件
pygame.display.update()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
```
运行该代码可以在窗口中看到一个光滑着色的三角形。其中,计算重心坐标的部分使用了重心坐标插值的方法,可以实现比较平滑的着色效果。
相关推荐
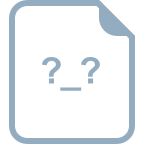
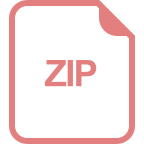
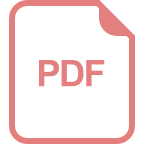














