写出一个可以下五子棋的java代码
时间: 2024-03-26 21:36:05 浏览: 16
以下是一个简单的可以下五子棋的 Java 代码,使用 Swing GUI 库:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Gomoku {
private JButton[][] buttons;
private int currentPlayer;
public Gomoku() {
JFrame frame = new JFrame("五子棋");
frame.setSize(600, 600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel board = new JPanel(new GridLayout(15, 15));
buttons = new JButton[15][15];
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 15; j++) {
JButton button = new JButton();
button.setPreferredSize(new Dimension(30, 30));
button.addActionListener(new ButtonListener(i, j));
board.add(button);
buttons[i][j] = button;
}
}
frame.getContentPane().add(board);
frame.setVisible(true);
currentPlayer = 1;
}
private void makeMove(int row, int col) {
if (buttons[row][col].getText().equals("")) {
if (currentPlayer == 1) {
buttons[row][col].setText("●");
} else {
buttons[row][col].setText("○");
}
if (checkWin(row, col)) {
JOptionPane.showMessageDialog(null, "Player " + currentPlayer + " wins!");
System.exit(0);
}
currentPlayer = 3 - currentPlayer; // 切换玩家
}
}
private boolean checkWin(int row, int col) {
String symbol = (currentPlayer == 1) ? "●" : "○";
// 检查行
int count = 0;
for (int i = 0; i < 15; i++) {
if (buttons[row][i].getText().equals(symbol)) {
count++;
if (count == 5) return true;
} else {
count = 0;
}
}
// 检查列
count = 0;
for (int i = 0; i < 15; i++) {
if (buttons[i][col].getText().equals(symbol)) {
count++;
if (count == 5) return true;
} else {
count = 0;
}
}
// 检查对角线
count = 0;
for (int i = -4; i <= 4; i++) {
int r = row + i;
int c = col + i;
if (r >= 0 && r < 15 && c >= 0 && c < 15 && buttons[r][c].getText().equals(symbol)) {
count++;
if (count == 5) return true;
} else {
count = 0;
}
}
// 检查反对角线
count = 0;
for (int i = -4; i <= 4; i++) {
int r = row + i;
int c = col - i;
if (r >= 0 && r < 15 && c >= 0 && c < 15 && buttons[r][c].getText().equals(symbol)) {
count++;
if (count == 5) return true;
} else {
count = 0;
}
}
return false;
}
private class ButtonListener implements ActionListener {
private int row, col;
public ButtonListener(int row, int col) {
this.row = row;
this.col = col;
}
public void actionPerformed(ActionEvent e) {
makeMove(row, col);
}
}
public static void main(String[] args) {
new Gomoku();
}
}
```
这个代码创建了一个 15x15 的五子棋棋盘,玩家可以通过点击按钮下棋子。程序会自动检查是否有五个棋子连成一线,如果有就弹出胜利提示。
相关推荐
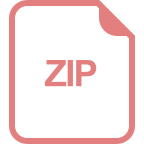
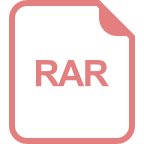
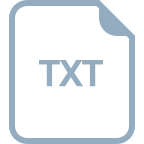














