Gram矩阵在风格迁移中的使用python代码
时间: 2023-12-03 18:43:21 浏览: 46
Gram矩阵是风格迁移中一个非常重要的概念,用于描述图片的纹理特征。下面是使用Python代码计算Gram矩阵的示例:
``` python
import tensorflow as tf
import numpy as np
import PIL.Image
# 加载图片并进行预处理
image_path = 'path/to/image.jpg'
image = np.array(PIL.Image.open(image_path))
image = tf.keras.applications.vgg19.preprocess_input(image)
# 加载VGG19模型,提取卷积层特征
model = tf.keras.applications.VGG19(include_top=False, weights='imagenet')
model.trainable = False
outputs = [layer.output for layer in model.layers]
feature_extractor = tf.keras.Model(inputs=model.inputs, outputs=outputs)
features = feature_extractor(image)
# 计算Gram矩阵
def gram_matrix(input_tensor):
result = tf.linalg.einsum('bijc,bijd->bcd', input_tensor, input_tensor)
input_shape = tf.shape(input_tensor)
num_locations = tf.cast(input_shape[1]*input_shape[2], tf.float32)
return result/(num_locations)
style_features = features[2:]
style_gram_matrices = [gram_matrix(feature) for feature in style_features]
```
在上面的示例代码中,我们首先加载了一张图片,并使用VGG19模型对其进行预处理和特征提取。然后,我们定义了一个函数 `gram_matrix` 来计算输入张量的Gram矩阵。最后,我们计算了图片的卷积层特征,并使用 `gram_matrix` 函数计算了每个特征的Gram矩阵。
需要注意的是,上面的示例代码中使用了TensorFlow 2.x版本的API,如果您使用的是TensorFlow 1.x版本,请将 `tf.linalg.einsum` 改为 `tf.einsum`,并将 `tf.keras.Model` 改为 `tf.compat.v1.keras.Model`。
相关推荐
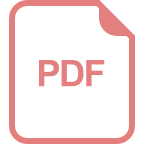
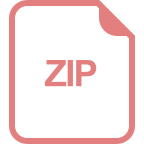













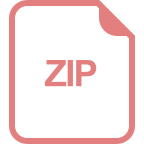