用Python实现斗地主人机对战
时间: 2023-11-05 17:51:28 浏览: 49
斗地主是一种比较常见的纸牌游戏,可以用Python编写一个基本的人机对战程序。
下面是一个简单的实现示例:
```python
import random
# 初始化牌组
cards = []
for i in range(3, 11):
cards.extend([str(i)] * 4)
cards.extend(['J'] * 4)
cards.extend(['Q'] * 4)
cards.extend(['K'] * 4)
cards.extend(['A'] * 4)
cards.extend(['2', '小王', '大王'])
# 洗牌
random.shuffle(cards)
# 定义牌型
card_types = {
'single': 1,
'pair': 2,
'triple': 3,
'triple_with_single': 4,
'triple_with_pair': 5,
'four_with_two_single': 6,
'four_with_two_pair': 7,
'straight': 8,
'straight_pair': 9,
'aircraft_with_wings': 10
}
# 牌型判断函数
def get_card_type(card_list):
card_len = len(card_list)
if card_len == 1:
return 'single'
elif card_len == 2 and card_list[0] == card_list[1]:
return 'pair'
elif card_len == 3 and card_list[0] == card_list[1] and card_list[1] == card_list[2]:
return 'triple'
elif card_len == 4 and card_list[0] == card_list[1] and card_list[1] == card_list[2] and card_list[2] == card_list[3]:
return 'four_with_two_single'
elif card_len == 5 and card_list[0] == card_list[1] and card_list[1] == card_list[2] and card_list[2] == card_list[3] and card_list[3] != card_list[4]:
return 'triple_with_single'
elif card_len == 5 and card_list[0] != card_list[1] and card_list[1] == card_list[2] and card_list[2] == card_list[3] and card_list[3] == card_list[4]:
return 'triple_with_single'
elif card_len == 6 and card_list[0] == card_list[1] and card_list[1] == card_list[2] and card_list[2] == card_list[3] and card_list[3] == card_list[4] and card_list[4] == card_list[5]:
return 'four_with_two_pair'
elif card_len >= 5 and card_len % 2 == 1:
return None
elif card_len >= 6 and card_list[-1] == '2':
return None
elif card_len == 6 and card_list[0] == card_list[1] and card_list[1] == card_list[2] and card_list[3] == card_list[4] and card_list[4] == card_list[5]:
return 'straight_pair'
elif card_len >= 5 and card_list[-1] != '2':
for i in range(card_len - 1):
if int(card_list[i + 1]) - int(card_list[i]) != 1:
return None
return 'straight'
else:
return None
# 定义出牌函数
def play_cards(hand_cards, last_cards=[]):
if not last_cards:
# 首次出牌,出单张牌
return [hand_cards.pop(0)]
else:
last_type = get_card_type(last_cards)
hand_type = get_card_type(hand_cards)
if hand_type is None or (last_type is not None and card_types[hand_type] < card_types[last_type]):
# 不能出牌或者手牌牌型不大于上家
return []
elif hand_type != last_type:
# 牌型不同,只能出炸弹或者火箭
if hand_type == 'four_with_two_single' or hand_type == 'four_with_two_pair':
return hand_cards[:4]
elif hand_type == 'aircraft_with_wings':
triple_list = []
for i in range(len(hand_cards) - 2):
if hand_cards[i] == hand_cards[i + 1] and hand_cards[i + 1] == hand_cards[i + 2]:
triple_list.append(hand_cards[i])
return triple_list * 3
else:
return []
else:
# 牌型相同,出牌逻辑
if hand_type == 'single' or hand_type == 'pair' or hand_type == 'triple':
for card in hand_cards:
if card > last_cards[0]:
return [card]
return []
elif hand_type == 'triple_with_single':
triple_list = []
for i in range(len(hand_cards) - 2):
if hand_cards[i] == hand_cards[i + 1] and hand_cards[i + 1] == hand_cards[i + 2]:
triple_list.append(hand_cards[i])
if len(triple_list) == 0:
return []
for triple in triple_list:
if triple > last_cards[0]:
single_list = [card for card in hand_cards if card != triple]
return [triple] + single_list[:1]
return []
elif hand_type == 'triple_with_pair':
triple_list = []
for i in range(len(hand_cards) - 2):
if hand_cards[i] == hand_cards[i + 1] and hand_cards[i + 1] == hand_cards[i + 2]:
triple_list.append(hand_cards[i])
if len(triple_list) == 0:
return []
for triple in triple_list:
if triple > last_cards[0]:
pair_list = [card for card in hand_cards if card != triple]
pair_list = [pair_list[i:i+2] for i in range(0, len(pair_list), 2)]
pair_list = sorted(pair_list, key=lambda x: int(x[0]))
return [triple] + pair_list[:1][0]
return []
elif hand_type == 'straight':
hand_len = len(hand_cards)
last_len = len(last_cards)
if hand_len != last_len:
return []
if hand_cards[-1] <= last_cards[-1]:
return []
for i in range(last_len):
if hand_cards[i] <= last_cards[i]:
return []
return hand_cards
elif hand_type == 'straight_pair':
hand_len = len(hand_cards)
last_len = len(last_cards)
if hand_len != last_len:
return []
if hand_cards[-1] <= last_cards[-1]:
return []
for i in range(last_len):
if hand_cards[i] <= last_cards[i]:
return []
pair_list = [hand_cards[i:i+2] for i in range(0, hand_len, 2)]
return pair_list
elif hand_type == 'four_with_two_single':
if hand_cards[0] <= last_cards[0]:
return []
return hand_cards[:4] + hand_cards[-2:]
elif hand_type == 'four_with_two_pair':
if hand_cards[0] <= last_cards[0]:
return []
pair_list = [hand_cards[i:i+2] for i in range(0, len(hand_cards), 2)]
pair_list = sorted(pair_list, key=lambda x: int(x[0]))
return hand_cards[:4] + pair_list[:2][0]
elif hand_type == 'aircraft_with_wings':
hand_len = len(hand_cards)
last_len = len(last_cards)
if hand_len != last_len:
return []
if hand_cards[-1] <= last_cards[-1]:
return []
triple_list = []
for i in range(len(hand_cards) - 2):
if hand_cards[i] == hand_cards[i + 1] and hand_cards[i + 1] == hand_cards[i + 2]:
triple_list.append(hand_cards[i])
if len(triple_list) != last_len // 4:
return []
triple_list = sorted(triple_list, key=lambda x: int(x))
wings_list = [card for card in hand_cards if card not in triple_list]
if len(wings_list) != last_len // 2:
return []
wings_list = [wings_list[i:i+(last_len // 4)] for i in range(0, len(wings_list), last_len // 4)]
return triple_list * 3 + wings_list[:1][0]
else:
return []
# 定义游戏函数
def play_game():
# 初始化玩家手牌
player1_cards = cards[:17]
player2_cards = cards[17:34]
player3_cards = cards[34:]
# 首次出牌
last_cards = []
turn = 1
while player1_cards and player2_cards and player3_cards:
if turn == 1:
print('玩家1出牌')
print('上一家出牌:', last_cards)
print('手牌:', player1_cards)
play_cards_list = play_cards(player1_cards, last_cards)
if not play_cards_list:
print('玩家1不出')
else:
print('玩家1出牌:', play_cards_list)
for card in play_cards_list:
player1_cards.remove(card)
last_cards = play_cards_list
turn = 2
elif turn == 2:
print('玩家2出牌')
print('上一家出牌:', last_cards)
print('手牌:', player2_cards)
play_cards_list = play_cards(player2_cards, last_cards)
if not play_cards_list:
print('玩家2不出')
else:
print('玩家2出牌:', play_cards_list)
for card in play_cards_list:
player2_cards.remove(card)
last_cards = play_cards_list
turn = 3
elif turn == 3:
print('玩家3出牌')
print('上一家出牌:', last_cards)
print('手牌:', player3_cards)
play_cards_list = play_cards(player3_cards, last_cards)
if not play_cards_list:
print('玩家3不出')
else:
print('玩家3出牌:', play_cards_list)
for card in play_cards_list:
player3_cards.remove(card)
last_cards = play_cards_list
turn = 1
if not player1_cards:
print('玩家1获胜')
elif not player2_cards:
print('玩家2获胜')
else:
print('玩家3获胜')
# 运行游戏
play_game()
```
这个程序实现了基本的斗地主人机对战逻辑,可以运行并进行游戏。
相关推荐
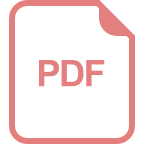
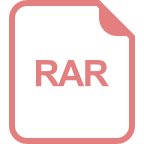
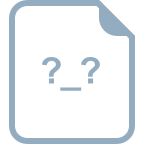














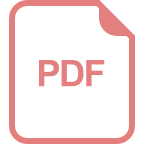