python车道线检测代码
时间: 2023-11-04 11:59:33 浏览: 176
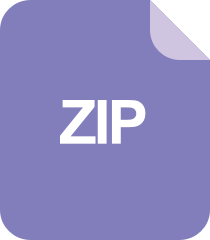
车道线检测代码 python
Python车道线检测代码可以使用OpenCV库实现。以下是一份Python车道线检测代码示例:
```
import cv2
import numpy as np
# 定义ROI区域
def region_of_interest(img, vertices):
mask = np.zeros_like(img)
match_mask_color = 255
cv2.fillPoly(mask, vertices, match_mask_color)
masked_image = cv2.bitwise_and(img, mask)
return masked_image
# 定义Hough变换参数
def hough_lines(img, rho, theta, threshold, min_line_len, max_line_gap):
lines = cv2.HoughLinesP(img, rho, theta, threshold, np.array([]), minLineLength=min_line_len, maxLineGap=max_line_gap)
line_img = np.zeros((img.shape[0], img.shape[1], 3), dtype=np.uint8)
draw_lines(line_img, lines)
return line_img
# 绘制车道线
def draw_lines(img, lines, color=[255, 0, 0], thickness=2):
left_lines, right_lines = [], []
for line in lines:
for x1, y1, x2, y2 in line:
k = (y2 - y1) / (x2 - x1)
if k < 0:
left_lines.append(line)
else:
right_lines.append(line)
if len(left_lines) > 0:
left_avg = np.average(left_lines, axis=0)
cv2.line(img, (int(left_avg[0][0]), int(left_avg[0][1])), (int(left_avg[0][2]), int(left_avg[0][3])), color, thickness)
if len(right_lines) > 0:
right_avg = np.average(right_lines, axis=0)
cv2.line(img, (int(right_avg[0][0]), int(right_avg[0][1])), (int(right_avg[0][2]), int(right_avg[0][3])), color, thickness)
# 读取图片
image = cv2.imread('test.jpg')
# 转为灰度图
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 高斯滤波
kernel_size = 5
blur_gray = cv2.GaussianBlur(gray, (kernel_size, kernel_size), 0)
# Canny边缘检测
low_threshold = 50
high_threshold = 150
edges = cv2.Canny(blur_gray, low_threshold, high_threshold)
# ROI区域选择
imshape = image.shape
vertices = np.array([[(0,imshape[0]),(450, 320), (550, 320), (imshape[1],imshape[0])]], dtype=np.int32)
masked_edges = region_of_interest(edges, vertices)
# Hough变换检测车道线
rho = 2
theta = np.pi / 180
threshold = 15
min_line_len = 60
max_line_gap = 30
line_image = hough_lines(masked_edges, rho, theta, threshold, min_line_len, max_line_gap)
# 结果叠加到原图上
result = cv2.addWeighted(image, 0.8, line_image, 1, 0)
# 显示结果
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
此代码可以检测车道线并绘制出车道线,运行结果会显示在窗口中。你可以自己更改代码中的参数来适配不同的图片。
阅读全文
相关推荐
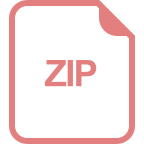

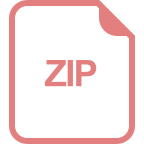
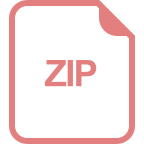
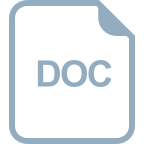
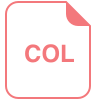
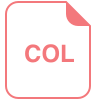
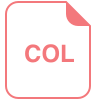
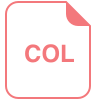
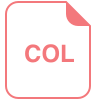
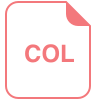
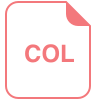
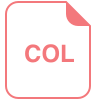
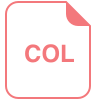
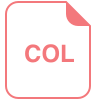

