如何在给定的一堆字母里找到目标字母的个数,用C++和C语言实现
时间: 2024-10-27 09:10:37 浏览: 17
在C++或C语言中,你可以通过遍历字符串数组或者字符数组来计算目标字母的数量。这里是一个简单的示例:
```cpp
#include <iostream>
#include <string>
// C++函数示例
int countCharInString(const std::string& str, char target) {
int count = 0;
for (char c : str) {
if (c == target)
count++;
}
return count;
}
// C语言函数示例
int count_char_in_string(char* str, char target, size_t len) {
int count = 0;
for (size_t i = 0; i < len; i++) {
if (str[i] == target)
count++;
}
return count;
}
int main() {
// 使用示例
std::string stringExample = "Hello, World!";
char targetChar = 'o';
// C++版本
int countCpp = countCharInString(stringExample, targetChar);
std::cout << "Target '" << targetChar << "' appears " << countCpp << " times in the string.\n";
// C版本
char cExample[] = "Hello, World!";
int countC = count_char_in_string(cExample, targetChar, sizeof(cExample) - 1); // 注意减去1以排除'\0'
printf("Target '%c' appears %d times in the string.\n", targetChar, countC);
return 0;
}
```
在这个例子中,`countCharInString` 和 `count_char_in_string` 分别是C++和C语言中的函数,它们接收一个字符串(或字符数组)以及一个目标字符,然后遍历整个字符串来计数目标字符的出现次数。
阅读全文
相关推荐


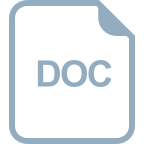

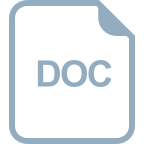













