C语言将稀疏阵采用十字链表压缩完整代码
时间: 2024-11-06 16:15:24 浏览: 15
在C语言中,十字链表是一种常见的用于表示稀疏矩阵的数据结构。这种数据结构通过存储非零元素及其位置,有效地节省了空间,特别适合于那些大部分元素为0的稀疏矩阵。下面是一个简单的示例代码,展示了如何使用十字链表来存储和操作稀疏矩阵:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int row, col; // 行和列索引
int value; // 值
struct Node* next[2]; // 每个节点有两个指向前一个行或列元素的指针
} SparseMatrixNode;
struct SparseMatrix {
SparseMatrixNode** data;
int rows, cols;
};
// 创建一个新的稀疏矩阵
SparseMatrix* createSparseMatrix(int rows, int cols) {
SparseMatrix* sm = (SparseMatrix*)malloc(sizeof(SparseMatrix));
sm->rows = rows;
sm->cols = cols;
sm->data = (SparseMatrixNode**)calloc(rows, sizeof(SparseMatrixNode*));
for (int i = 0; i < rows; ++i)
sm->data[i] = (SparseMatrixNode*)calloc(cols, sizeof(SparseMatrixNode));
return sm;
}
// 插入元素到矩阵
void insert(SparseMatrix* sm, int row, int col, int value) {
SparseMatrixNode* newNode = (SparseMatrixNode*)malloc(sizeof(SparseMatrixNode));
newNode->row = row;
newNode->col = col;
newNode->value = value;
newNode->next[0] = sm->data[row][col];
newNode->next[1] = sm->data[row + 1][col]; // 如果下一个单元格存在
if (newNode->row == sm->rows - 1 || sm->data[newNode->row + 1][col] == NULL) { // 列尾或不存在
newNode->next[1] = NULL;
}
sm->data[row][col] = newNode;
}
// 打印矩阵
void printSparseMatrix(SparseMatrix* sm) {
for (int i = 0; i < sm->rows; ++i) {
for (int j = 0; j < sm->cols; ++j) {
SparseMatrixNode* node = sm->data[i][j];
while (node != NULL) {
printf("(%d,%d): %d ", i, j, node->value);
node = node->next[j % 2]; // 遍历同一行或同一列的节点
}
}
printf("\n");
}
}
// 示例
int main() {
SparseMatrix* sm = createSparseMatrix(5, 5);
insert(sm, 0, 0, 1); // 插入元素
insert(sm, 1, 2, 2); // 插入更多元素
printSparseMatrix(sm);
free(sm->data[0]);
free(sm->data);
free(sm);
return 0;
}
```
这个示例展示了基本的十字链表稀疏矩阵操作,包括创建、插入元素以及打印。请注意,实际应用中需要添加错误处理和内存管理。
阅读全文
相关推荐
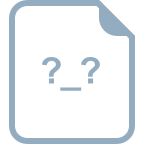
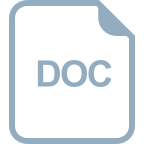




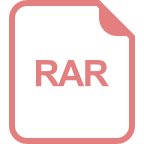
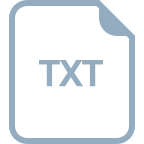
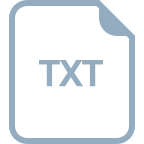
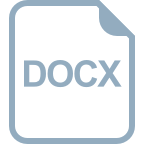
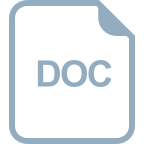
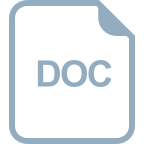
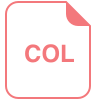




