用python写一个程序实现如下功能,长方形小车沿着贝塞尔曲线切线方向运动,要求可视化动态显示,小车可以用长方形替代显示
时间: 2024-04-16 21:24:57 浏览: 152
当然可以!你可以使用Python的matplotlib库来实现可视化动态显示,并使用numpy库来进行贝塞尔曲线的计算。下面是一个简单的示例程序,演示了小车沿着贝塞尔曲线切线方向运动的效果:
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
def bezier_curve(points, t):
n = len(points) - 1
result = np.zeros_like(points[0])
for i in range(n + 1):
result += binomial_coefficient(n, i) * ((1 - t) ** (n - i)) * (t ** i) * points[i]
return result
def binomial_coefficient(n, k):
return np.math.factorial(n) / (np.math.factorial(k) * np.math.factorial(n - k))
def update(frame):
t = frame / frames
tangent = bezier_curve_derivative(points, t)
angle = np.arctan2(tangent[1], tangent[0])
car.set_transform(plt.transforms.Affine2D().rotate(angle).translated(positions[frame][0], positions[frame][1]))
return car,
# 贝塞尔曲线控制点
points = np.array([[0, 0], [1, 1], [2, -1], [3, 0]])
# 计算贝塞尔曲线上的点
frames = 100
positions = [bezier_curve(points, t) for t in np.linspace(0, 1, frames)]
# 计算贝塞尔曲线切线方向
bezier_curve_derivative = np.gradient(positions, axis=0)
# 创建画布和轴
fig, ax = plt.subplots()
ax.set_xlim(-2, 4)
ax.set_ylim(-2, 2)
# 创建小车
car = plt.Rectangle((0, 0), 0.2, 0.1, fc='blue')
ax.add_patch(car)
# 动画更新函数
animation = FuncAnimation(fig, update, frames=frames, interval=50, blit=True)
plt.show()
```
在这个示例程序中,你可以根据需要修改贝塞尔曲线的控制点(points),以及调整动画的帧数(frames)和小车的形状(car)。运行程序后,会显示一个动态的窗口,其中小车沿着贝塞尔曲线切线方向运动。
请确保你已经安装了matplotlib和numpy库,可以使用以下命令进行安装:
```
pip install matplotlib numpy
```
希望这可以帮助到你!如有其他问题,请随时提问。
阅读全文
相关推荐
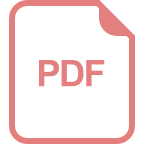













