使用C++编写一个3*3矩阵类,通过运算符重载的方式实现矩阵功能:矩阵相乘运算(*)、矩阵相加运算(+)、相等判断运算(==)、赋值运算(=)。每种矩阵功能写出3个测试用例。
时间: 2024-09-10 09:09:25 浏览: 55
首先,我们创建一个名为`Matrix`的类,包含3x3矩阵的元素数组以及一些必要的成员函数。然后我们将通过重载运算符实现矩阵的相乘、相加、相等判断和赋值操作。以下是相应的代码:
```cpp
#include <iostream>
#include <vector>
class Matrix {
private:
std::vector<std::vector<int>> data;
int rows, cols;
public:
Matrix(int r, int c) : rows(r), cols(c) { data.resize(r, std::vector<int>(c)); }
// 运算符重载
Matrix operator+(const Matrix& other) const {
if (rows != other.cols) throw std::runtime_error("Incompatible matrix sizes for addition");
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i)
for (int j = 0; j < cols; ++j)
result.data[i][j] = data[i][j] + other.data[i][j];
return result;
}
Matrix operator*(const Matrix& other) const {
if (cols != other.rows) throw std::runtime_error("Incompatible matrix sizes for multiplication");
Matrix result(rows, other.cols);
for (int i = 0; i < rows; ++i)
for (int j = 0; j < other.cols; ++j)
for (int k = 0; k < cols; ++k)
result.data[i][j] += data[i][k] * other.data[k][j];
return result;
}
bool operator==(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) return false;
for (int i = 0; i < rows; ++i)
for (int j = 0; j < cols; ++j)
if (data[i][j] != other.data[i][j])
return false;
return true;
}
Matrix& operator=(const Matrix& other) {
if (this == &other) return *this;
rows = other.rows;
cols = other.cols;
data = other.data;
return *this;
}
};
// 测试用例
int main() {
Matrix m1(3, 3);
m1.data = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
Matrix m2(3, 3);
m2.data = {{9, 8, 7}, {6, 5, 4}, {3, 2, 1}};
// 矩阵相加
Matrix sum = m1 + m2;
std::cout << "Sum of m1 and m2:\n";
for (auto row : sum.data)
for (int val : row)
std::cout << val << " ";
std::cout << "\n";
// 矩阵相乘
try {
Matrix product = m1 * m2;
std::cout << "Product of m1 and m2:\n";
for (auto row : product.data)
for (int val : row)
std::cout << val << " ";
std::cout << "\n";
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
// 相等判断
if (m1 == m2) {
std::cout << "m1 and m2 are equal.\n";
} else {
std::cout << "m1 and m2 are not equal.\n";
}
// 赋值
m1 = m2;
std::cout << "After assignment:\n";
for (auto row : m1.data)
for (int val : row)
std::cout << val << " ";
std::cout << "\n";
return 0;
}
```
在这段代码中,我们测试了矩阵相加、相乘、相等检查和赋值功能。注意,由于矩阵乘法的特性,我们还需要处理大小兼容性错误。
阅读全文
相关推荐
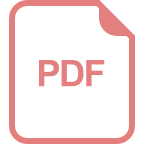
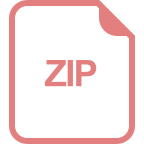
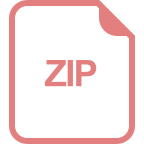















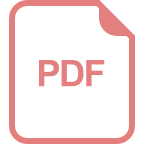