单链表实现集合A和集合B的并和交。头插法创建单链表LA,尾插法创建单链表LB,然后实现集合的两种运算,结果保存在单链表里面并输出结果。给出必要的提示。
时间: 2024-10-23 20:07:49 浏览: 18
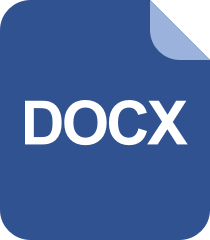
利用带头结点的单链表实现两个集合的并、交、差运算.docx
要实现单链表A和B的并集以及交集,我们需要先定义链表节点的数据结构,包括数据元素和指向下一个节点的指针。这里我假设数据元素都是可比较的(如整数或字符串)。我们将使用头插法(在链表头部插入元素)创建`LA`和尾插法(在链表尾部插入元素)创建`LB`。
首先,定义链表节点结构体:
```c
typedef struct Node {
int data; // 数据域
struct Node* next; // 指向下一个节点的指针
} Node;
```
接下来,我们需要两个辅助函数:插入到链表头部和尾部:
```c
// 头插法插入节点
void insertAtHead(Node** head, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = *head;
*head = newNode;
}
// 尾插法插入节点
void insertAtTail(Node** tail, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
if (*tail != NULL) {
(*tail)->next = newNode;
} else {
*tail = newNode;
}
}
```
现在我们有了链表操作的基础,我们可以开始实现并集和交集:
1. 并集操作(`union`):
- 遍历链表A,每个元素都添加到新链表中
- 如果链表B有相同元素,也添加到新链表中
```c
// 并集操作
void unionSet(Node* la, Node* lb, Node** result) {
Node* currentLa = la;
while (currentLa != NULL) {
// 插入链表A中的元素到结果链表
insertAtHead(result, currentLa->data);
currentLa = currentLa->next;
// 如果链表B也有该元素,也加入结果
Node* currentLb = lb;
while (currentLb != NULL) {
if (currentLa->data == currentLb->data) {
insertAtHead(result, currentLa->data); // 只需添加一次
}
currentLb = currentLb->next;
}
}
}
```
2. 交集操作(`intersection`):
- 遍历链表A,如果元素也在链表B中,则添加到结果链表
```c
// 交集操作
void intersectionSet(Node* la, Node* lb, Node** result) {
Node* currentLa = la;
Node* currentLb = lb;
while (currentLa != NULL && currentLb != NULL) {
if (currentLa->data == currentLb->data) {
insertAtHead(result, currentLa->data);
currentLa = currentLa->next;
currentLb = currentLb->next;
} else if (currentLa->data < currentLb->data) {
currentLa = currentLa->next;
} else {
currentLb = currentLb->next;
}
}
}
```
最后,创建链表`LA`、`LB`并执行并集和交集操作后,遍历结果链表并打印元素。
阅读全文
相关推荐
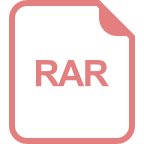
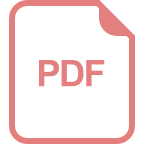
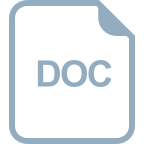














