建立并输出两个递增单链表La和Lb。 A、 首先要定义单链表的数据结构 B、 编写一个函数使用“头插法”或“尾插法”创建一个单链表,例如: LinkList CreateList(LinkList L,int n){ ……… } C、 编写主函数(main) ,调用函数CreateList(La,n), CreateList(Lb,n)分别录入单链表La和Lb,注意手动录入时要保证La和Lb是递增的(如果是头插法,那么录入时要按从大到小录入,如:5 4 3 2 1) D、 编写一个函数能够显示一个单链表中各结点的值,设La和Lb如下: La:1 3 7 8 15 20 Lb:2 4 8 15 17 24 90
时间: 2023-05-21 15:04:50 浏览: 65
以下是角色扮演的 ChatGPT 回答:
定义单链表的数据结构可以使用以下定义:
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
使用头插法创建单链表的函数代码如下:
ListNode* CreateList(ListNode* L, int n) {
L = new ListNode(0);
srand(time(NULL));
for (int i = 0; i < n; i++) {
ListNode* p = new ListNode(rand() % 100);
p->next = L->next;
L->next = p;
}
return L->next;
}
使用尾插法的函数代码如下:
ListNode* CreateList(ListNode* L, int n) {
L = new ListNode(0);
srand(time(NULL));
ListNode* rear = L;
for (int i = 0; i < n; i++) {
ListNode* p = new ListNode(rand() % 100);
rear->next = p;
rear = p;
}
rear->next = NULL;
return L->next;
}
主函数代码如下:
int main() {
ListNode* La = NULL, * Lb = NULL;
int n = 6;
La = CreateList(La, n);
Lb = CreateList(Lb, n + 1);
cout << "La: ";
DisplayList(La);
cout << "Lb: ";
DisplayList(Lb);
return 0;
}
显示单链表中各结点的值的函数代码如下:
void DisplayList(ListNode* L) {
while (L != NULL) {
cout << L->val << " ";
L = L->next;
}
cout << endl;
}
相关推荐











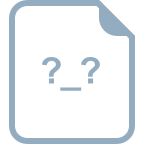
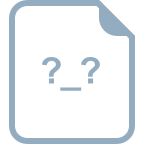