用c实现rsa算法加解密代码
时间: 2024-09-23 13:14:34 浏览: 79
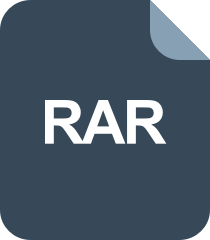
RSA.rar_RSA算法Python_python实现加密_rsa加密_加密_加密解密

RSA(Rivest-Shamir-Adleman)是一种非对称加密算法,常用于数据传输的安全保护。在C语言中实现RSA加密和解密涉及生成大数、模指数运算、密钥生成等步骤。这里是一个简化的示例,仅展示核心部分,实际应用需要考虑安全性和错误处理:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// RSA关键参数和辅助函数省略
// 加密函数 (大数乘法)
int rsa_encrypt(int m, int e, int n) {
int result = 1;
for (; m > 0; m >>= 1, e = e * e % n) {
if (m & 1)
result = (result * e) % n;
}
return result;
}
// 解密函数 (欧几里得逆元)
int rsa_decrypt(int c, int d, int n) {
int i = 0, x = 0, y = n - 1;
while (y != 0) {
int q = x / y;
int t = y;
y = x % y;
x = t;
t = d - q * i;
d = i;
i = t;
}
return c * (int)(pow((double)d, (double)n - 2) + 0.5); // 四舍五入防止溢出
}
int main() {
// 省略密钥生成和加载过程
int plaintext = ...; // 待加密的明文
int ciphertext = rsa_encrypt(plaintext, public_e, public_n);
printf("Encrypted message: %d\n", ciphertext);
int decrypted_text = rsa_decrypt(ciphertext, private_d, private_n);
printf("Decrypted message: %d\n", decrypted_text);
return 0;
}
```
阅读全文
相关推荐
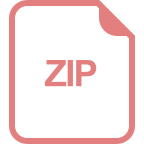
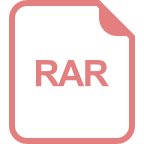















