使用简洁的c++语言完整实现对RSA加解密算法的完整代码
时间: 2024-10-09 18:07:37 浏览: 41
在C++中,RSA加密算法通常涉及大整数运算,因此我们通常会利用一些库如`<openssl>`来处理,因为它已经包含了必要的大数支持和安全的加密功能。以下是使用OpenSSL库实现RSA加解密的一个简化示例:
```cpp
#include <iostream>
#include <string>
#include <openssl/pem.h>
#include <openssl/rsa.h>
std::string encrypt(const std::string& message, RSA* rsa) {
unsigned char encrypted[EVP_MAX_BLOCK_SIZE];
int len;
if (RSA_public_encrypt(message.size(), reinterpret_cast<const unsigned char*>(message.c_str()), encrypted, rsa, RSA_PKCS1_OAEP_PADDING)) {
len = static_cast<int>(strlen(reinterpret_cast<char*>(encrypted)));
return std::string(reinterpret_cast<char*>(encrypted), len);
} else {
throw std::runtime_error("Encryption failed");
}
}
std::string decrypt(const std::string& ciphertext, RSA* rsa) {
unsigned char decrypted[EVP_MAX_BLOCK_SIZE];
int len;
if (RSA_private_decrypt(ciphertext.size(), reinterpret_cast<const unsigned char*>(ciphertext.c_str()), decrypted, rsa, RSA_PKCS1_OAEP_PADDING)) {
len = static_cast<int>(strlen(reinterpret_cast<char*>(decrypted)));
return std::string(reinterpret_cast<char*>(decrypted), len);
} else {
throw std::runtime_error("Decryption failed");
}
}
int main() {
// 实例化RSA公钥和私钥
RSA *pub_key = nullptr, *priv_key = nullptr;
BIGNUM *n, *e, *d, *p, *q; // 大数变量
// ... 加载公钥和私钥到OpenSSL上下文中 ...
// 使用公钥加密
std::string plaintext = "Hello, World!";
std::string encrypted_text = encrypt(plaintext, pub_key);
// 使用私钥解密
std::string decrypted_text = decrypt(encrypted_text, priv_key);
// 输出结果
std::cout << "Original Text: " << plaintext << "\n";
std::cout << "Encrypted Text: " << encrypted_text << "\n";
std::cout << "Decrypted Text: " << decrypted_text << "\n";
// 清理并释放资源
RSA_free(pub_key);
RSA_free(priv_key);
BN_free(n);
BN_free(e);
BN_free(d);
BN_free(p);
BN_free(q);
return 0;
}
```
请注意,这只是一个基本的示例,实际应用中你需要处理PEM文件格式、错误检查以及加载/保存密钥等步骤。此外,由于RSA加密的复杂性,建议在生产环境中使用成熟的加密库,而不是自行实现。
阅读全文
相关推荐
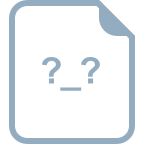
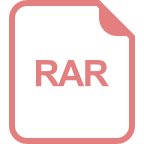
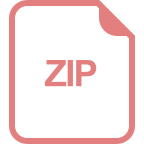
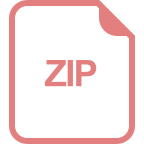
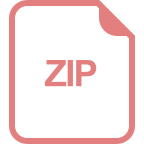
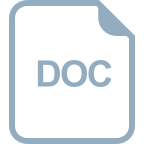
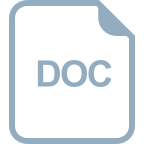
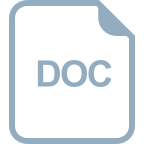
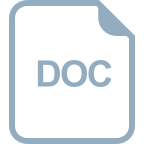
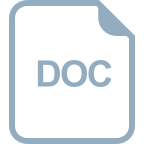
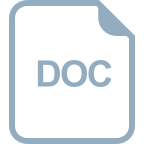
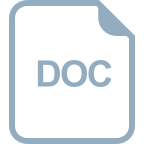
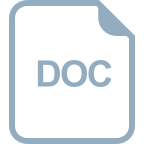





