corpus =list(words.tolist()) ^^^^^^^^^^^^ AttributeError: 'generator' object has no attribute 'tolist'
时间: 2023-11-13 12:43:06 浏览: 47
这个错误是因为你尝试对一个生成器对象调用 `tolist()` 方法,而生成器对象没有 `tolist()` 方法。`tolist()` 方法通常是用于将数组或矩阵转换为列表的方法。
要解决这个问题,你可以考虑将生成器对象转换为列表对象,然后再调用 `tolist()` 方法。你可以使用 `list()` 函数将生成器对象转换为列表对象,然后再调用 `tolist()` 方法。
下面是一个示例代码:
```python
corpus = list(words)
corpus = np.array(corpus)
corpus = corpus.tolist()
```
在这个示例中,我们首先将生成器对象 `words` 转换为列表对象 `corpus`,然后将列表对象转换为 NumPy 数组 `corpus`,最后使用 `tolist()` 方法将数组转换回列表。请根据你的具体情况进行适当的修改。
相关问题
AttributeError: 'Tokenizer' object has no attribute 'transform'
`AttributeError: 'Tokenizer' object has no attribute 'transform'` 是一个Python错误信息,表示在尝试调用`Tokenizer`对象的`transform`属性时出现了问题。这个错误通常发生在使用`Tokenizer`对象时,尝试调用一个不存在的方法或属性。
`Tokenizer`是`sklearn.feature_extraction.text`模块中的一个类,用于将文本数据转换为特征向量。然而,`Tokenizer`类并没有名为`transform`的方法。如果你想要对文本数据进行转换,你应该使用`CountVectorizer`或`TfidfVectorizer`等类。
以下是一个简单的例子,展示了如何使用`CountVectorizer`对文本数据进行转换:
```python
from sklearn.feature_extraction.text import CountVectorizer
# 示例文本数据
corpus = [
'这是第一个文档',
'这是第二个文档',
'这是第三个文档'
]
# 创建CountVectorizer对象
vectorizer = CountVectorizer()
# 使用fit_transform方法对文本数据进行转换
X = vectorizer.fit_transform(corpus)
# 输出转换后的特征向量
print(X.toarray())
```
在这个例子中,我们首先从`sklearn.feature_extraction.text`模块导入`CountVectorizer`类。然后,我们创建一个包含三个文档的文本数据列表。接下来,我们创建一个`CountVectorizer`对象,并使用`fit_transform`方法对文本数据进行转换。最后,我们将转换后的特征向量输出到控制台。
AttributeError: type object 'Word2Vec' has no attribute 'Text8Corpus'
AttributeError: type object 'Word2Vec' has no attribute 'Text8Corpus' 这个错误通常是由于gensim库的版本不兼容引起的。gensim库在较新的版本中已经将Text8Corpus方法更名为corpora.Text8Corpus,所以在较新的gensim版本中,应该使用corpora.Text8Corpus代替Text8Corpus。你可以尝试更新gensim库到最新版本,然后将代码中的Text8Corpus替换为corpora.Text8Corpus。这样应该可以解决这个错误。
相关推荐
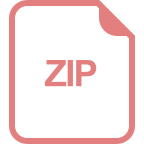













