使用c语言编写程序,要求利用栈实现算术表达式求值,利用栈实现后缀表达式的计算,仅需要实现一位数值(0-9)的+,-,*,/,()等 运算
时间: 2024-10-27 19:05:25 浏览: 31
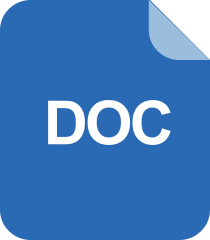
c语言 实现二叉树操作 用栈实现算术表达式求值
在C语言中,可以使用栈来实现后缀表达式(逆波兰表示法,Reverse Polish Notation,RPN)的计算。以下是一个简单的实现过程:
1. **中缀表达式转后缀表达式** (前缀表达式更合适,因为后缀表达式是RPN)
- 创建一个栈,遍历中缀表达式,当遇到左括号时,压入;遇到操作数,直接压入;遇到右括号时,将栈顶的元素弹出并依次放在新字符串中,直到遇到左括号为止。
2. **后缀表达式求值**
- 初始化一个整型栈,用于存放操作数。
- 再次遍历后缀表达式,当遇到数字时,压入栈;遇到操作符时,从栈中弹出两个操作数执行相应运算,然后将结果压回栈。
- 遍历结束后,栈顶的唯一元素即为表达式的结果。
下面是一个简单的C语言代码片段:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_TOKENS 100
int isOperator(char op) {
return op == '+' || op == '-' || op == '*' || op == '/';
}
int precedence(char op) {
return (op == '*' || op == '/') ? 2 : (op == '+' || op == '-') ? 1 : 0;
}
int popTwoOperands(int *stack, int &top) {
if (top < 2) return -1;
int a = stack[top--], b = stack[top--];
return a, b;
}
double evaluatePostfix(char *postfix) {
int stack[MAX_TOKENS], top = 0;
for (char c : postfix) {
if (isdigit(c))
stack[top++] = c - '0';
else {
int a, b;
if ((a = popTwoOperands(stack, top)) == -1) return -1;
switch (c) {
case '+':
stack[top++] = a + b;
break;
case '-':
stack[top++] = a - b;
break;
case '*':
stack[top++] = a * b;
break;
case '/':
if (b != 0)
stack[top++] = a / b;
else
return -1;
break;
}
}
}
return stack[0];
}
int main() {
char infix[] = "1+2*3-4";
char postfix[100];
// 转换过程省略...
printf("Result: %.2f\n", evaluatePostfix(postfix));
return 0;
}
```
阅读全文
相关推荐
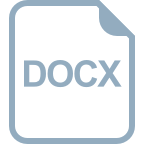
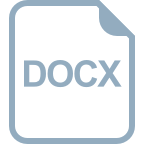
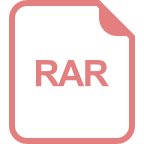
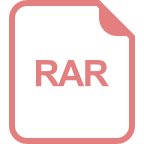
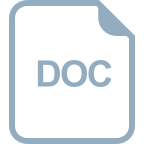






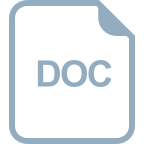
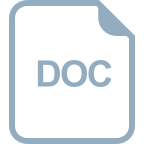




