用c语言写出简单的算术表达式中可能出现运算数、运算符、圆括号0,从键盘输人一个表达式,实现表达式的求值,并输出结果.
时间: 2024-12-06 09:33:38 浏览: 11
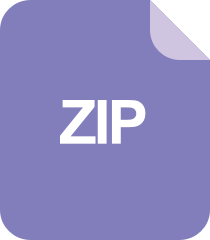
数据结构大作业C++实现简单的计算器——算术表达式计算(包含实验报告)

在C语言中,你可以使用递归函数和栈来处理这个需求。这里是一个简单的例子,它会读取用户输入的算术表达式并计算其值:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h> // 包含字符处理函数
// 定义操作数和操作符的枚举
typedef enum { NUMBER, OPERATOR } Token;
// 结构体表示表达式树节点
typedef struct Node {
Token type;
union {
double num; // 如果是数字
char op; // 如果是运算符
};
struct Node* left, *right; // 子节点指针
} Node;
// 函数用于获取下一个token
Node* getNextToken(char* input) {
while (isspace(*input)) input++;
if (*input == '\0') return NULL; // 表示结束
if (isdigit(*input)) {
Node* node = malloc(sizeof(Node));
node->type = NUMBER;
node->num = atof(input);
input += sizeof(double); // 跳过数字部分
return node;
} else if (*input == '+' || *input == '-' || *input == '*' || *input == '/') {
Node* node = malloc(sizeof(Node));
node->type = OPERATOR;
node->op = *input;
input++; // 跳过运算符
return node;
} else if (*input == '(') {
Node* node = malloc(sizeof(Node));
node->type = '(';
input++; // 跳过左括号
return node;
} else if (*input == ')') {
Node* node = malloc(sizeof(Node));
node->type = ')';
input++; // 跳过右括号
return node;
} else {
printf("Invalid character: %c\n", *input);
return NULL;
}
}
// 根据运算符计算表达式值
double calculate(Node* node) {
switch (node->type) {
case NUMBER:
return node->num;
case '+':
return calculate(node->left) + calculate(node->right);
case '-':
return calculate(node->left) - calculate(node->right);
case '*':
return calculate(node->left) * calculate(node->right);
case '/':
if (calculate(node->right) != 0)
return calculate(node->left) / calculate(node->right);
else
printf("Error: Division by zero!\n");
default:
return 0; // 非法操作符,返回0
}
}
int main() {
char input[100];
printf("Enter an arithmetic expression: ");
fgets(input, sizeof(input), stdin);
// 删除字符串末尾的换行符
input[strlen(input) - 1] = '\0';
Node* root = getNextToken(input);
if (!root) {
printf("Invalid expression\n");
return 1;
}
double result = calculate(root);
printf("Result: %.2f\n", result);
return 0;
}
```
运行程序后,用户可以输入像 "5+3*(4-2)" 这样的表达式,然后程序将计算并显示结果。
阅读全文
相关推荐
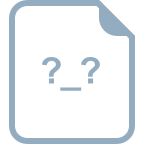
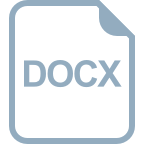
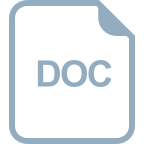


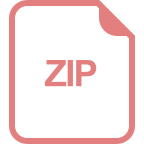
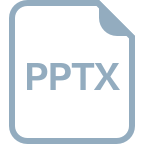
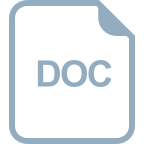
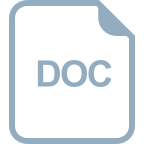
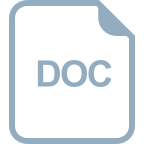
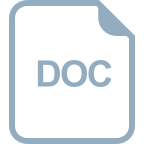






