算术表达式有前缀表示法、中缀表示法和后缀表示法等形式。日常使用的算术表达式是采用中缀表示法,即二元运算符位于两个运算数中间。请设计程序将中缀表达式转换为后缀表达式。用C语言实现
时间: 2024-10-14 21:00:27 浏览: 88
在C语言中,将中缀表达式转换为后缀表达式也称为逆波兰表示(Reverse Polish Notation,RPN),通常会通过栈的数据结构来实现。这里是一个简单的步骤描述:
1. 创建一个空栈和一个结果数组。
2. 遍历中缀表达式的每个字符:
- 如果遇到数字,直接添加到结果数组。
- 如果遇到运算符,按照优先级处理:
a. 先进栈:如果当前运算符的优先级低于栈顶运算符,则将栈顶运算符压入结果数组,并弹出栈顶元素。
b. 后进:如果当前运算符的优先级高于或等于栈顶运算符,或栈为空,则将当前运算符压入栈。
3. 当遍历完所有字符后,栈中剩余的运算符依次压入结果数组。
4. 结果数组就是后缀表达式。
下面是一个简化的C语言函数示例,用于转换单个操作数的加减乘除:
```c
#include <stdio.h>
#include <stdlib.h>
char* infixToPostfix(char* infix) {
stack_t *s = (stack_t*)malloc(sizeof(stack_t));
char postfix[100];
int i, j = 0;
const char* operators = "+-*/";
for (i = 0; infix[i] != '\0'; i++) {
if (isdigit(infix[i])) { // 数字直接加入后缀
postfix[j++] = infix[i];
} else if (strchr(operators, infix[i]) != NULL) { // 运算符
while (s->top && strchr(operators, s->top) && precedence(s->top) >= precedence(infix[i])) {
postfix[j++] = s->top;
s->top = s->data[s->size - 1]; // 弹出栈顶元素
s->size--;
}
s->top = infix[i]; // 将运算符压入栈
} else if (infix[i] == ' ') { // 忽略空格
continue;
}
}
while (s->top) {
postfix[j++] = s->top;
s->top = s->data[s->size - 1];
s->size--;
}
postfix[j] = '\0';
free(s->data);
free(s);
return postfix;
}
// 辅助函数,用于比较运算符的优先级
int precedence(char op) {
switch (op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
// 示例用法
int main() {
char infix[] = "A+B*C";
printf("Infix: %s\n", infix);
char* postfix = infixToPostfix(infix);
printf("Postfix: %s\n", postfix);
return 0;
}
```
请注意,这个示例仅适用于最简单的四则运算。实际应用中,还需要处理括号以及更复杂的表达式。完整的实现可能需要更复杂的算法,例如Shunting Yard算法。
阅读全文
相关推荐
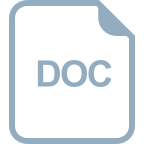





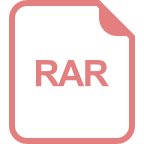









