unity实现不同端口接收不同数据
时间: 2024-05-04 17:19:45 浏览: 18
在 Unity 中实现不同端口接收不同数据可以通过使用不同的 Socket 对象来实现。以下是一个基本的示例代码:
```csharp
using System.Net;
using System.Net.Sockets;
public class SocketServer : MonoBehaviour
{
public int port1 = 1234;
public int port2 = 5678;
private Socket socket1;
private Socket socket2;
void Start()
{
// 创建 Socket 对象并绑定到指定端口
socket1 = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
socket1.Bind(new IPEndPoint(IPAddress.Any, port1));
socket2 = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
socket2.Bind(new IPEndPoint(IPAddress.Any, port2));
// 开始异步接收数据
StartReceive(socket1);
StartReceive(socket2);
}
private void StartReceive(Socket socket)
{
EndPoint endPoint = new IPEndPoint(IPAddress.Any, 0);
byte[] buffer = new byte[1024];
socket.BeginReceiveFrom(buffer, 0, buffer.Length, SocketFlags.None, ref endPoint, ReceiveCallback, socket);
}
private void ReceiveCallback(IAsyncResult result)
{
Socket socket = (Socket)result.AsyncState;
EndPoint endPoint = new IPEndPoint(IPAddress.Any, 0);
byte[] buffer = new byte[1024];
int length = socket.EndReceiveFrom(result, ref endPoint);
// 根据不同的端口处理接收到的数据
if (((IPEndPoint)endPoint).Port == port1)
{
// 处理 port1 接收到的数据
}
else if (((IPEndPoint)endPoint).Port == port2)
{
// 处理 port2 接收到的数据
}
// 继续异步接收数据
StartReceive(socket);
}
}
```
在上面的示例代码中,我们创建了两个不同的 Socket 对象并分别绑定到不同的端口上。然后通过异步接收数据的方式来监听这两个端口,当接收到数据时,根据不同的端口来处理接收到的数据。最后,再次调用异步接收数据的方法来继续监听这两个端口。
相关推荐
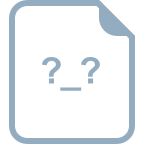
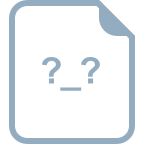
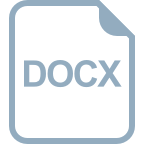
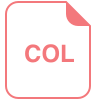
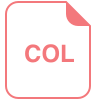












