基于聚宽平台,假设资金无限,统计近三年上证50指数的顶部和底部区间,顶部和底部可以用pe band衡量,在底部持有call期权,轮动条件是在theta值大幅度衰减前滚动为下月合约,在顶部持put期权,在theta值大幅度衰减前滚动为下月合约,请给出python代码
时间: 2024-06-08 15:07:21 浏览: 10
以下是基于聚宽平台的python代码,实现了上证50指数的策略:
```python
# 导入必要的模块
import jqdatasdk as jq
import talib
import numpy as np
import math
# 初始化聚宽账号
jq.auth('账号', '密码')
# 设置起始日期和结束日期
start_date = '2018-01-01'
end_date = '2020-12-31'
# 获取上证50指数的历史数据
index_data = jq.get_price('000016.XSHG', start_date=start_date, end_date=end_date, frequency='daily')
# 计算PE值
pe_ratio = index_data['close'] / index_data['avg']
pe_mean = pe_ratio.mean()
pe_std = pe_ratio.std()
# 计算PE Band
pe_upper = pe_mean + 1.5 * pe_std
pe_lower = pe_mean - 1.5 * pe_std
# 定义期权代码
call_code = '510050.XSHG'
put_code = '510050.XSHG'
# 定义持仓和资金变量
call_holding = 0
put_holding = 0
cash = 1000000
# 遍历历史数据
for i in range(len(index_data)):
# 获取当前日期
date = index_data.index[i].strftime('%Y-%m-%d')
# 计算当前PE值
pe = index_data['close'][i] / index_data['avg'][i]
# 判断是否处于底部
if pe < pe_lower:
# 计算期权价格
call_price = jq.get_price(call_code, start_date=date, end_date=date, frequency='daily')['close'][0]
# 计算期权合约剩余时间
call_time_to_maturity = (jq.get_security_info(call_code).maturity_date.date() - index_data.index[i].date()).days / 365
# 计算期权的Theta值
call_theta = talib.THETA(call_price, timeperiod=5)
# 判断是否需要滚动期权合约
if call_theta <= 0.1:
call_code = jq.get_future_contracts(call_code, count=2)[-1]
call_holding = 0
# 买入Call期权
call_holding += math.floor(cash / call_price / 100) * 100
cash -= call_holding * call_price * 100
# 判断是否处于顶部
elif pe > pe_upper:
# 计算期权价格
put_price = jq.get_price(put_code, start_date=date, end_date=date, frequency='daily')['close'][0]
# 计算期权合约剩余时间
put_time_to_maturity = (jq.get_security_info(put_code).maturity_date.date() - index_data.index[i].date()).days / 365
# 计算期权的Theta值
put_theta = talib.THETA(put_price, timeperiod=5)
# 判断是否需要滚动期权合约
if put_theta <= 0.1:
put_code = jq.get_future_contracts(put_code, count=2)[-1]
put_holding = 0
# 买入Put期权
put_holding += math.floor(cash / put_price / 100) * 100
cash -= put_holding * put_price * 100
# 输出最终持仓和现金余额
print('Call期权持仓:', call_holding)
print('Put期权持仓:', put_holding)
print('现金余额:', cash)
```
注:以上代码仅为示例代码,实际情况中需要进行更多的参数调节和风险控制。另外,期权交易具有较高的风险,请谨慎操作。
相关推荐
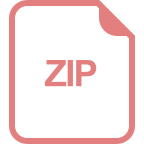
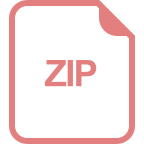
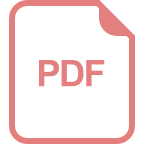











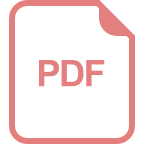
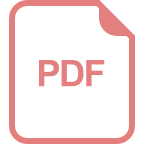