python写一个空心圆形
时间: 2023-03-30 17:03:01 浏览: 137
您好,以下是 Python 代码,可以绘制一个空心圆形:
```python
import turtle
# 设置画笔
pen = turtle.Turtle()
pen.pensize(2)
pen.color("black", "white")
pen.speed()
# 绘制圆形
pen.penup()
pen.goto(, -100)
pen.pendown()
pen.begin_fill()
pen.circle(100)
pen.end_fill()
# 绘制空心圆形
pen.penup()
pen.goto(, -80)
pen.pendown()
pen.color("black", "black")
pen.begin_fill()
pen.circle(80)
pen.end_fill()
# 隐藏画笔
pen.hideturtle()
# 显示绘图窗口
turtle.done()
```
希望对您有所帮助。
相关问题
如何用python编写一个空心圆形
### 回答1:
可以使用matplotlib库中的matplotlib.patches.Circle函数来绘制空心圆。例如:import matplotlib.pyplot as plt
x, y = 0.0, 0.0
radius = 2.0
circle = plt.Circle((x,y), radius, fill=False)
ax = plt.gca()
ax.add_patch(circle)
plt.axis('scaled')
plt.show()
### 回答2:
要用Python编写一个空心圆形,我们可以使用turtle库来实现。Turtle库是Python的一个绘图库,可以帮助我们在图形窗口上进行绘图。
首先,我们需要导入turtle库,并创建一个turtle对象:
```python
import turtle
t = turtle.Turtle()
```
然后,我们可以使用turtle库中的circle函数来绘制一个填充圆形。然而,我们需要实现一个空心圆形,所以我们需要调整一下绘图的参数。
我们可以将填充设置为透明的,然后将笔的宽度设置为我们希望的线宽。然后我们可以使用circle函数来绘制一个实心圆。
```python
t.fillcolor("transparent")
t.pensize(3)
t.circle(100)
```
接下来,我们需要将笔移动到空心圆形的起始位置,实现空心效果。可以使用penup函数将笔抬起,然后使用setpos函数将笔移动到圆形的起始位置。
```python
t.penup()
t.setposition(0, -100)
```
最后,我们需要将笔重新放下,开始绘制一个内部为空的空心圆形。可以使用pendown函数将笔放下,然后使用circle函数来绘制一个较小的空心圆,使外部的圆形边缘为空心。
```python
t.pendown()
t.circle(80)
```
完成上述步骤后,我们可以调用turtle库中的done函数,来关闭图形窗口。
```python
turtle.done()
```
以上就是用Python编写一个空心圆形的步骤。通过设置填充为透明,笔的宽度和使用circle函数的调用,我们可以实现一个内部为空的空心圆形。
### 回答3:
编写一个空心圆形的方法是使用Python的turtle库来绘制图形。以下是绘制空心圆形的代码:
```python
import turtle
# 创建一个Turtle对象
pen = turtle.Turtle()
# 设置画笔颜色和宽度
pen.color("black")
pen.pensize(3)
# 移动画笔到起始位置
pen.penup()
pen.goto(0, -100)
pen.pendown()
# 设置填充颜色
pen.fillcolor("white")
# 绘制一个空心圆形
pen.begin_fill()
pen.circle(100) # 绘制一个半径为100的圆
pen.end_fill()
# 隐藏画笔
pen.hideturtle()
# 关闭turtle绘图窗口
turtle.done()
```
以上代码会在窗口中显示一个空心的白色圆形,圆形的半径为100像素。你可以根据需要调整代码中的圆形半径、颜色和画笔宽度等参数。
Python pgzurn 怎么画空心圆
Python 的 `pgzrun` 是一个基于 Pygame 的模块,它简化了游戏开发流程。如果你想在 pgzrun 中画空心圆,你可以使用 Pygame 的内建函数 `pygame.draw.circle()` 结合一些额外步骤来实现。这里是一个简单的示例:
```python
import pygame
# 初始化 Pygame
pygame.init()
# 设置窗口大小
size = (800, 600)
screen = pygame.display.set_mode(size)
# 定义颜色
fill_color = (255, 0, 0) # 红色填充色
outline_color = (0, 0, 255) # 蓝色边线色
# 创建一个圆形矩形区域,用于计算半径
circle_area = pygame.Rect(100, 100, 200, 200)
# 计算圆心坐标
center = circle_area.center
# 绘制空心圆
pygame.draw.rect(screen, fill_color, circle_area, 0) # 全部填充红色
pygame.draw.circle(screen, outline_color, center, circle_area.width // 2, 4) # 在中心画出蓝色实线边框,宽度为半径的一半,线条粗细为4像素
# 更新屏幕
pygame.display.flip()
# 游戏主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 清理并退出
pygame.quit()
```
在这个例子中,我们先创建了一个红色的矩形填充整个圆形区域,然后在圆心处绘制一个蓝色的圆环作为边框。`draw.circle()` 的第四个参数表示边框的宽度。
阅读全文
相关推荐
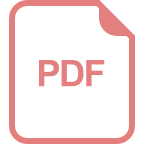
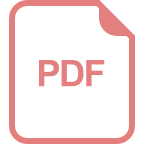












