多目标优化遗传算法完整代码
时间: 2024-04-26 11:19:36 浏览: 236
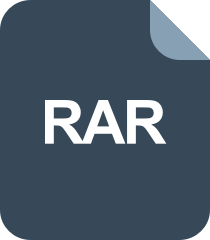
多目标遗传算法(代码完整,数据齐全)
多目标优化遗传算法(MOGA)是一种用于解决多目标优化问题的进化算法。下面是一个简单的MOGA的完整代码示例:
```matlab
% 设置参数
popSize = 100; % 种群大小
maxGen = 100; % 最大迭代次数
numObj = 2; % 目标函数数量
% 初始化种群
pop = rand(popSize, numObj); % 随机生成种群
% 迭代进化
for gen = 1:maxGen
% 计算适应度
fitness = calculateFitness(pop);
% 选择操作
selectedPop = selection(pop, fitness);
% 交叉操作
crossedPop = crossover(selectedPop);
% 变异操作
mutatedPop = mutation(crossedPop);
% 合并新种群和原种群
combinedPop = [pop; mutatedPop];
% 非支配排序
[fronts, ranks] = nonDominatedSort(combinedPop);
% 拥挤度计算
crowdingDistances = crowdingDistance(combinedPop, fronts);
% 选择下一代种群
pop = nextGeneration(combinedPop, fronts, ranks, crowdingDistances, popSize);
end
% 输出最终结果
paretoFront = pop(ranks == 1, :); % 获取帕累托前沿解集合
disp(paretoFront);
% 计算适应度函数(根据具体问题进行定义)
function fitness = calculateFitness(population)
% 这里假设有两个目标函数,可以根据具体问题进行修改
fitness(:, 1) = population(:, 1).^2 + population(:, 2).^2;
fitness(:, 2) = (population(:, 1)-1).^2 + population(:, 2).^2;
end
% 选择操作(这里使用锦标赛选择)
function selectedPop = selection(population, fitness)
tournamentSize = 2; % 锦标赛大小
popSize = size(population, 1);
selectedPop = zeros(size(population));
for i = 1:popSize
% 随机选择锦标赛参与者
participants = randperm(popSize, tournamentSize);
% 选择最优个体
[~, winnerIndex] = min(fitness(participants, :));
selectedPop(i, :) = population(participants(winnerIndex), :);
end
end
% 交叉操作(这里使用单点交叉)
function crossedPop = crossover(selectedPop)
crossoverRate = 0.8; % 交叉概率
popSize = size(selectedPop, 1);
numObj = size(selectedPop, 2);
crossedPop = zeros(size(selectedPop));
for i = 1:2:popSize
% 随机选择两个个体进行交叉
if rand < crossoverRate
crossoverPoint = randi(numObj-1);
crossedPop(i, :) = [selectedPop(i, 1:crossoverPoint), selectedPop(i+1, crossoverPoint+1:end)];
crossedPop(i+1, :) = [selectedPop(i+1, 1:crossoverPoint), selectedPop(i, crossoverPoint+1:end)];
else
crossedPop(i, :) = selectedPop(i, :);
crossedPop(i+1, :) = selectedPop(i+1, :);
end
end
end
% 变异操作(这里使用高斯变异)
function mutatedPop = mutation(crossedPop)
mutationRate = 0.1; % 变异概率
popSize = size(crossedPop, 1);
numObj = size(crossedPop, 2);
mutatedPop = crossedPop;
for i = 1:popSize
% 对每个个体的每个基因进行变异
for j = 1:numObj
if rand < mutationRate
mutatedPop(i, j) = crossedPop(i, j) + randn;
end
end
end
end
% 非支配排序
function [fronts, ranks] = nonDominatedSort(population)
popSize = size(population, 1);
numObj = size(population, 2);
fronts = cell(popSize, 1);
ranks = zeros(popSize, 1);
dominatedCount = zeros(popSize, 1);
dominatedSet = cell(popSize, 1);
for i = 1:popSize
fronts{i} = [];
dominatedSet{i} = [];
for j = 1:popSize
if i == j
continue;
end
if all(population(j,:) <= population(i,:)) && any(population(j,:) < population(i,:))
fronts{i} = [fronts{i}, j];
elseif all(population(i,:) <= population(j,:)) && any(population(i,:) < population(j,:))
dominatedCount(i) = dominatedCount(i) + 1;
dominatedSet{i} = [dominatedSet{i}, j];
end
end
if dominatedCount(i) == 0
ranks(i) = 1;
end
end
frontIndex = 1;
while ~isempty(fronts{frontIndex})
nextFront = [];
for i = fronts{frontIndex}
for j = dominatedSet{i}
dominatedCount(j) = dominatedCount(j) - 1;
if dominatedCount(j) == 0
ranks(j) = frontIndex + 1;
nextFront = [nextFront, j];
end
end
end
frontIndex = frontIndex + 1;
fronts{frontIndex} = nextFront;
end
end
% 拥挤度计算
function crowdingDistances = crowdingDistance(population, fronts)
popSize = size(population, 1);
numObj = size(population, 2);
crowdingDistances = zeros(popSize, 1);
for i = 1:length(fronts)
front = fronts{i};
for j = 1:numObj
[~, sortedIndices] = sort(population(front, j));
crowdingDistances(front(sortedIndices(1))) = inf;
crowdingDistances(front(sortedIndices(end))) = inf;
for k = 2:length(front)-1
crowdingDistances(front(sortedIndices(k))) = crowdingDistances(front(sortedIndices(k))) + ...
(population(front(sortedIndices(k+1)), j) -1)), j)) / ...
(max(population(front, j)) - min(population(front, j)));
end
end
end
end
% 选择下一代种群
function nextGenPop = nextGeneration(population, fronts, ranks, crowdingDistances, popSize)
popSize = size(population, 1);
numObj = size(population, 2);
nextGenPop = zeros(popSize, numObj);
nextGenIndex = 1;
frontIndex = 1;
while nextGenIndex <= popSize
front = fronts{frontIndex};
if nextGenIndex + length(front) <= popSize
nextGenPop(nextGenIndex:nextGenIndex+length(front)-1, :) = population(front, :);
nextGenIndex = nextGenIndex + length(front);
else
[~, sortedIndices] = sort(crowdingDistances(front), 'descend');
nextGenPop(nextGenIndex:popSize, :) = population(front(sortedIndices(1:popSize-nextGenIndex+1)), :);
break;
end
frontIndex = frontIndex + 1;
end
end
```
这段代码实现了一个简单的MOGA,其中包括初始化种群、迭代进化、适应度计算、选择操作、交叉操作、变异操作、非支配排序、拥挤度计算和选择下一代种群等步骤。你可以根据具体问题进行适当的修改和扩展。
希望对你有帮助!如果你有任何问题,请随时提问。
阅读全文
相关推荐
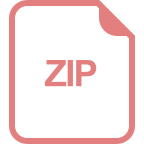
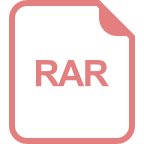
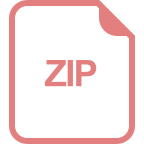
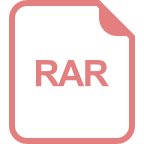
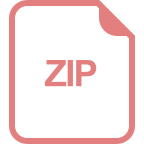
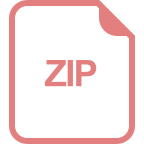
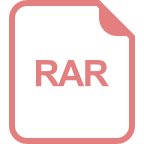
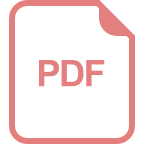
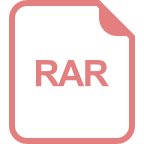
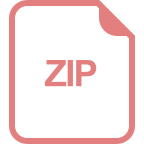
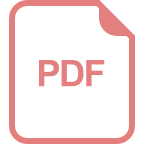
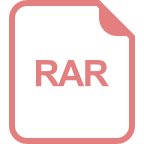
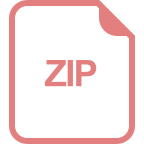
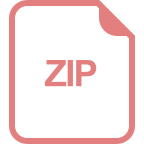