unity 遍历列表
时间: 2024-08-23 22:00:54 浏览: 43
在Unity中,遍历列表通常涉及到迭代数组、List<T> 或其他可枚举类型的元素。这里有几种常见的方法:
1. **For 循环**:对于List<T>等实现了IEnumerable接口的对象,最常见的是使用for循环,例如:
```csharp
List<int> myList = new List<int>{1, 2, 3};
for(int i = 0; i < myList.Count; i++)
{
Debug.Log(myList[i]);
}
```
2. **foreach 循环**:更推荐使用foreach,因为它会自动处理索引和迭代终止,更适合遍历内容,而不关心索引位置,例如:
```csharp
foreach(int num in myList)
{
Debug.Log(num);
}
```
3. **Linq查询**:如果要进行更复杂的过滤或操作,可以使用LINQ,如筛选特定条件的元素:
```csharp
var filteredList = myList.Where(x => x > 1);
foreach(var item in filteredList)
{
Debug.Log(item);
}
```
4. **迭代器(Iterator)**:对于自定义的迭代器对象(IEnumerator/IEnumerable),也可以通过类似的方式进行遍历,但这个场景相对较少见。
相关问题
unity 遍历Animation中所有动画名称
在Unity中,遍历`Animation`组件中所有动画名称通常通过脚本来完成,因为`Animation`组件本身并不直接提供获取所有动画名称的属性。你可以创建一个脚本,然后挂载到包含`Animation`的GameObject上,使用`Animator`类的方法来访问动画。
以下是一个简单的C#示例:
```csharp
using UnityEngine;
public class GetAnimations : MonoBehaviour
{
public Animation animation; // 假设已经给Animation组件赋值
void Start()
{
List<string> animations = new List<string>();
foreach (string animName in animation.runtimeAnimatorController.animationClips)
{
animations.Add(animName);
}
Debug.Log("Available animations:");
foreach (string anim in animations)
{
Debug.Log(anim);
}
}
}
```
在这个脚本中,我们首先创建了一个空列表`animations`,然后在`Start()`函数里遍历了`runtimeAnimatorController.animationClips`,这个集合包含了当前控制器的所有动画剪辑名称。最后,我们在控制台打印出所有的动画名。
unity 中遍历列表内的元素的方法
在Unity中,遍历列表或数组的元素可以使用`for`循环或`foreach`语句。以下是两种方法的示例代码:
使用`for`循环遍历列表:
```csharp
List<int> list = new List<int>() {1, 2, 3, 4, 5};
for (int i = 0; i < list.Count; i++) {
int element = list[i];
Debug.Log(element);
}
```
使用`foreach`语句遍历列表:
```csharp
List<int> list = new List<int>() {1, 2, 3, 4, 5};
foreach (int element in list) {
Debug.Log(element);
}
```
使用`for`循环遍历数组:
```csharp
int[] array = new int[] {1, 2, 3, 4, 5};
for (int i = 0; i < array.Length; i++) {
int element = array[i];
Debug.Log(element);
}
```
使用`foreach`语句遍历数组:
```csharp
int[] array = new int[] {1, 2, 3, 4, 5};
foreach (int element in array) {
Debug.Log(element);
}
```
需要注意的是,在使用`for`循环遍历列表或数组时,需要通过`Count`或`Length`属性来获取元素的数量,而在使用`foreach`语句遍历列表或数组时,不需要获取元素的数量。
阅读全文
相关推荐
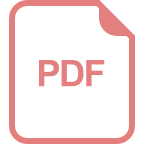
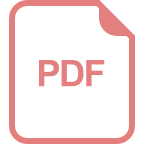
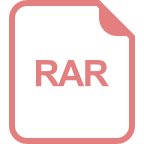













