unity 列表加载StreamingAssets文件夹里图片
时间: 2024-04-17 09:25:56 浏览: 189
要在Unity中加载`StreamingAssets`文件夹中的图片到列表中,你可以使用`System.IO`命名空间和Unity的UI系统。下面是一个示例代码:
```csharp
using UnityEngine;
using UnityEngine.UI;
using System.IO;
public class ImageListLoader : MonoBehaviour
{
public GameObject imagePrefab; // 图片预制体
public Transform content; // 列表的内容容器
private string imagesFolderPath = "Images"; // 图片文件夹路径
private void Start()
{
LoadImages();
}
private void LoadImages()
{
string streamingPath = Path.Combine(Application.streamingAssetsPath, imagesFolderPath);
if (Directory.Exists(streamingPath))
{
string[] imageFiles = Directory.GetFiles(streamingPath);
foreach (string imagePath in imageFiles)
{
// 加载图片预制体
GameObject imageGO = Instantiate(imagePrefab, content);
// 获取图片名称
string imageName = Path.GetFileNameWithoutExtension(imagePath);
// 设置图片预制体的名称
imageGO.name = imageName;
// 设置图片显示
Image imageComponent = imageGO.GetComponent<Image>();
StartCoroutine(LoadImageCoroutine(imagePath, imageComponent));
}
}
}
private IEnumerator LoadImageCoroutine(string imagePath, Image imageComponent)
{
var request = UnityEngine.Networking.UnityWebRequestTexture.GetTexture("file://" + imagePath);
yield return request.SendWebRequest();
if (request.result != UnityEngine.Networking.UnityWebRequest.Result.Success)
{
Debug.LogError("Failed to load image: " + request.error);
yield break;
}
Texture2D texture = ((UnityEngine.Networking.DownloadHandlerTexture)request.downloadHandler).texture;
Sprite sprite = Sprite.Create(texture, new Rect(0, 0, texture.width, texture.height), Vector2.one * 0.5f);
imageComponent.sprite = sprite;
}
}
```
这段代码假设你已经在场景中创建了一个空对象,并将`ImageListLoader`脚本附加到该对象上。你还需要创建一个图片预制体,并将其分配给`imagePrefab`变量。`content`变量引用了列表的内容容器,你可以在Unity编辑器中将容器对象指定为变量。
在`Start`方法中,我们调用`LoadImages`方法来加载图片。`LoadImages`方法首先构造了`StreamingAssets`文件夹中图片的路径,并检查路径是否存在。然后,我们使用`Directory.GetFiles`方法获取图片文件夹中的所有文件路径,并遍历这些文件路径。
在循环中,我们实例化一个图片预制体,并设置预制体的名称为图片名称(去除扩展名)。然后,我们获取预制体上的`Image`组件,并使用协程`LoadImageCoroutine`加载图片并设置到`Image`组件上。
在`LoadImageCoroutine`方法中,我们使用`UnityWebRequestTexture.GetTexture`方法加载图片。通过协程等待加载完成后,我们检查是否成功加载。如果成功加载,我们创建一个`Sprite`并设置到传入的`Image`组件上。
请确保你已经正确设置了场景和图片资源,并根据需要修改代码中的路径和加载的资源类型。同时,需要在列表的内容容器中创建一个空对象作为父级,并将其指定给脚本中的`content`变量。
阅读全文
相关推荐
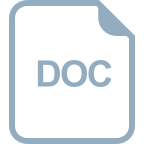
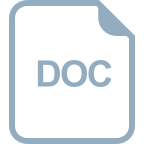
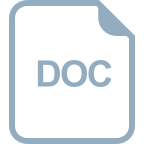















