二叉树的基本运算及其实现 编程实现:建立二叉树并输出下列二叉树的(1)结点个数;(2)叶子结点个数;(3)深度;(4)先序序列、中序序列和后序序列。
时间: 2023-06-11 22:06:03 浏览: 70
好的,我可以为您提供代码实现。首先,我们需要定义二叉树的结构体:
```c++
struct TreeNode {
int val; // 结点的值
TreeNode* left; // 左子树指针
TreeNode* right; // 右子树指针
TreeNode(int x) : val(x), left(nullptr), right(nullptr) {} // 构造函数
};
```
然后,我们可以通过递归的方式来建立二叉树:
```c++
TreeNode* buildTree() {
int val;
cin >> val; // 输入当前结点的值
if (val == -1) { // 如果值为 -1,表示当前结点为空
return nullptr;
}
TreeNode* root = new TreeNode(val); // 创建当前结点
root->left = buildTree(); // 递归建立左子树
root->right = buildTree(); // 递归建立右子树
return root;
}
```
接下来,我们可以实现求结点个数、叶子结点个数、深度以及先序、中序、后序遍历的函数:
```c++
int getNodeCount(TreeNode* root) {
if (root == nullptr) {
return 0;
}
return getNodeCount(root->left) + getNodeCount(root->right) + 1;
}
int getLeafCount(TreeNode* root) {
if (root == nullptr) {
return 0;
}
if (root->left == nullptr && root->right == nullptr) {
return 1;
}
return getLeafCount(root->left) + getLeafCount(root->right);
}
int getDepth(TreeNode* root) {
if (root == nullptr) {
return 0;
}
return max(getDepth(root->left), getDepth(root->right)) + 1;
}
void preorder(TreeNode* root) {
if (root == nullptr) {
return;
}
cout << root->val << " ";
preorder(root->left);
preorder(root->right);
}
void inorder(TreeNode* root) {
if (root == nullptr) {
return;
}
inorder(root->left);
cout << root->val << " ";
inorder(root->right);
}
void postorder(TreeNode* root) {
if (root == nullptr) {
return;
}
postorder(root->left);
postorder(root->right);
cout << root->val << " ";
}
```
最后,我们可以在主函数中调用上述函数:
```c++
int main() {
TreeNode* root = buildTree();
cout << "结点个数:" << getNodeCount(root) << endl;
cout << "叶子结点个数:" << getLeafCount(root) << endl;
cout << "深度:" << getDepth(root) << endl;
cout << "先序遍历:";
preorder(root);
cout << endl;
cout << "中序遍历:";
inorder(root);
cout << endl;
cout << "后序遍历:";
postorder(root);
cout << endl;
return 0;
}
```
完整代码如下:
阅读全文
相关推荐
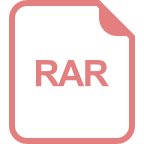
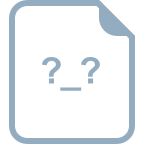












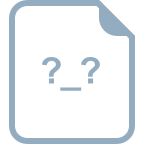