解析这段代码 def renderPeople(self, i, j): if self.direction == 'down': screen.blit(self.down_people, (j*35-7, i*35-27)) if self.direction == 'left': screen.blit(self.left_people, (j*35-7, i*35-27)) if self.direction == 'right': screen.blit(self.right_people, (j*35-7, i*35-27)) if self.direction == 'up': screen.blit(self.up_people, (j*35-7, i*35-27))
时间: 2024-03-26 09:37:35 浏览: 41
这段代码定义了一个方法名为`renderPeople`,它的作用是渲染游戏中角色的图像。具体实现过程如下:
首先,该方法接受两个参数`i`和`j`,代表角色所在的行和列。
然后,通过判断`self.direction`来确定角色的朝向,如果朝向是`down`,则将`down_people`图像渲染在屏幕上,坐标为`(j*35-7, i*35-27)`;如果朝向是`left`,则将`left_people`图像渲染在屏幕上,坐标为`(j*35-7, i*35-27)`;如果朝向是`right`,则将`right_people`图像渲染在屏幕上,坐标为`(j*35-7, i*35-27)`;如果朝向是`up`,则将`up_people`图像渲染在屏幕上,坐标为`(j*35-7, i*35-27)`。
最后,`screen.blit`方法将图像渲染在屏幕上,其中`screen`表示游戏窗口的Surface对象,`blit`是Surface对象的方法。
相关问题
t贪吃蛇小游戏代码python
以下是一个简单的贪吃蛇小游戏的 Python 代码示例:
```python
import pygame
import random
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义方向常量
UP = 0
DOWN = 1
LEFT = 2
RIGHT = 3
# 初始化 pygame
pygame.init()
# 设置窗口大小和标题
screen_width = 640
screen_height = 480
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("贪吃蛇小游戏")
# 设置时钟
clock = pygame.time.Clock()
# 加载字体
font = pygame.font.SysFont(None, 25)
# 定义贪吃蛇类
class Snake:
def __init__(self):
self.length = 1
self.positions = [((screen_width / 2), (screen_height / 2))]
self.direction = random.choice([UP, DOWN, LEFT, RIGHT])
self.color = GREEN
self.score = 0
def get_head_position(self):
return self.positions[0]
def turn(self, point):
if self.length > 1 and (point[0] * -1, point[1] * -1) == self.direction:
return
else:
self.direction = point
def move(self):
cur = self.get_head_position()
x, y = self.direction
new = (((cur[0] + (x * 10)) % screen_width), (cur[1] + (y * 10)) % screen_height)
if len(self.positions) > 2 and new in self.positions[2:]:
self.reset()
else:
self.positions.insert(0, new)
if len(self.positions) > self.length:
self.positions.pop()
def reset(self):
self.length = 1
self.positions = [((screen_width / 2), (screen_height / 2))]
self.direction = random.choice([UP, DOWN, LEFT, RIGHT])
self.score = 0
def draw(self, surface):
for p in self.positions:
r = pygame.Rect((p[0], p[1]), (10, 10))
pygame.draw.rect(surface, self.color, r)
pygame.draw.rect(surface, BLACK, r, 1)
def handle_keys(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
self.turn((0, -1))
elif event.key == pygame.K_DOWN:
self.turn((0, 1))
elif event.key == pygame.K_LEFT:
self.turn((-1, 0))
elif event.key == pygame.K_RIGHT:
self.turn((1, 0))
# 定义食物类
class Food:
def __init__(self):
self.position = (0, 0)
self.color = RED
self.randomize_position()
def randomize_position(self):
self.position = (random.randint(0, screen_width // 10 - 1) * 10, random.randint(0, screen_height // 10 - 1) * 10)
def draw(self, surface):
r = pygame.Rect((self.position[0], self.position[1]), (10, 10))
pygame.draw.rect(surface, self.color, r)
pygame.draw.rect(surface, BLACK, r, 1)
# 创建贪吃蛇和食物对象
snake = Snake()
food = Food()
# 游戏循环
while True:
# 处理事件
snake.handle_keys()
# 移动贪吃蛇
snake.move()
# 判断是否吃到食物
if snake.get_head_position() == food.position:
snake.length += 1
snake.score += 1
food.randomize_position()
# 绘制游戏界面
screen.fill(WHITE)
snake.draw(screen)
food.draw(screen)
text = font.render("Score: " + str(snake.score), True, BLACK)
screen.blit(text, (5, 10))
pygame.display.update()
# 控制帧率
clock.tick(10)
```
这个示例代码使用 Pygame 模块进行游戏开发,实现了基本的贪吃蛇小游戏功能。你可以根据需要进行修改和扩展。
帮我生成一段贪吃蛇代码,要求可以在Python3上运行
好的,以下是一段Python3的贪吃蛇代码:
```
import pygame
import random
# 初始化pygame
pygame.init()
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# 定义窗口大小
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 600
# 创建窗口
screen = pygame.display.set_mode([SCREEN_WIDTH, SCREEN_HEIGHT])
# 设置窗口标题
pygame.display.set_caption("Snake")
# 设置时钟对象
clock = pygame.time.Clock()
# 定义方块大小
BLOCK_SIZE = 20
# 定义字体
font = pygame.font.SysFont(None, 25)
# 定义蛇类
class Snake(pygame.sprite.Sprite):
def __init__(self, x, y):
super().__init__()
self.image = pygame.Surface([BLOCK_SIZE, BLOCK_SIZE])
self.image.fill(GREEN)
self.rect = self.image.get_rect()
self.rect.x = x
self.rect.y = y
# 定义食物类
class Food(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.Surface([BLOCK_SIZE, BLOCK_SIZE])
self.image.fill(RED)
self.rect = self.image.get_rect()
self.rect.x = random.randrange(0, SCREEN_WIDTH-BLOCK_SIZE, BLOCK_SIZE)
self.rect.y = random.randrange(0, SCREEN_HEIGHT-BLOCK_SIZE, BLOCK_SIZE)
# 定义主函数
def main():
# 初始化游戏
snake_list = pygame.sprite.Group()
all_sprites_list = pygame.sprite.Group()
food_list = pygame.sprite.Group()
snake = Snake(300, 300)
snake_list.add(snake)
all_sprites_list.add(snake)
food = Food()
food_list.add(food)
all_sprites_list.add(food)
# 定义初始方向
direction = 'right'
# 定义初始分数
score = 0
# 游戏循环
done = False
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
direction = 'left'
elif event.key == pygame.K_RIGHT:
direction = 'right'
elif event.key == pygame.K_UP:
direction = 'up'
elif event.key == pygame.K_DOWN:
direction = 'down'
# 移动蛇头
if direction == 'right':
snake.rect.x += BLOCK_SIZE
elif direction == 'left':
snake.rect.x -= BLOCK_SIZE
elif direction == 'up':
snake.rect.y -= BLOCK_SIZE
elif direction == 'down':
snake.rect.y += BLOCK_SIZE
# 如果蛇头碰到边界或者碰到自己,结束游戏
if snake.rect.x < 0 or snake.rect.y < 0 or snake.rect.x > SCREEN_WIDTH-BLOCK_SIZE or snake.rect.y > SCREEN_HEIGHT-BLOCK_SIZE or pygame.sprite.spritecollide(snake, snake_list, False):
done = True
# 如果蛇头碰到食物,分数加一,重新生成食物
if pygame.sprite.spritecollide(snake, food_list, True):
score += 1
food = Food()
food_list.add(food)
all_sprites_list.add(food)
# 删除蛇尾
if len(snake_list) > score:
snake_tail = snake_list.sprites()[0]
snake_list.remove(snake_tail)
all_sprites_list.remove(snake_tail)
# 添加蛇头
snake_list.add(snake)
all_sprites_list.add(snake)
# 清除屏幕
screen.fill(WHITE)
# 绘制所有精灵
all_sprites_list.draw(screen)
# 绘制分数
text = font.render("Score: {}".format(score), True, BLACK)
screen.blit(text, [10, 10])
# 更新屏幕
pygame.display.flip()
# 设置帧率
clock.tick(10)
# 退出pygame
pygame.quit()
# 调用主函数
if __name__ == "__main__":
main()
```
希望对你有帮助。
阅读全文
相关推荐
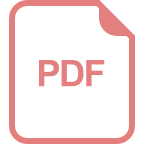














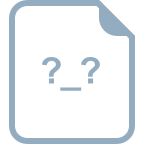